Software Services
For Companies
For Developers
Portfolio
Build With Us
Table of Contents:
Get Senior Engineers Straight To Your Inbox

Every month we send out our top new engineers in our network who are looking for work, be the first to get informed when top engineers become available

At Slashdev, we connect top-tier software engineers with innovative companies. Our network includes the most talented developers worldwide, carefully vetted to ensure exceptional quality and reliability.
Build With Us
How To Ace Your Laravel Interview/
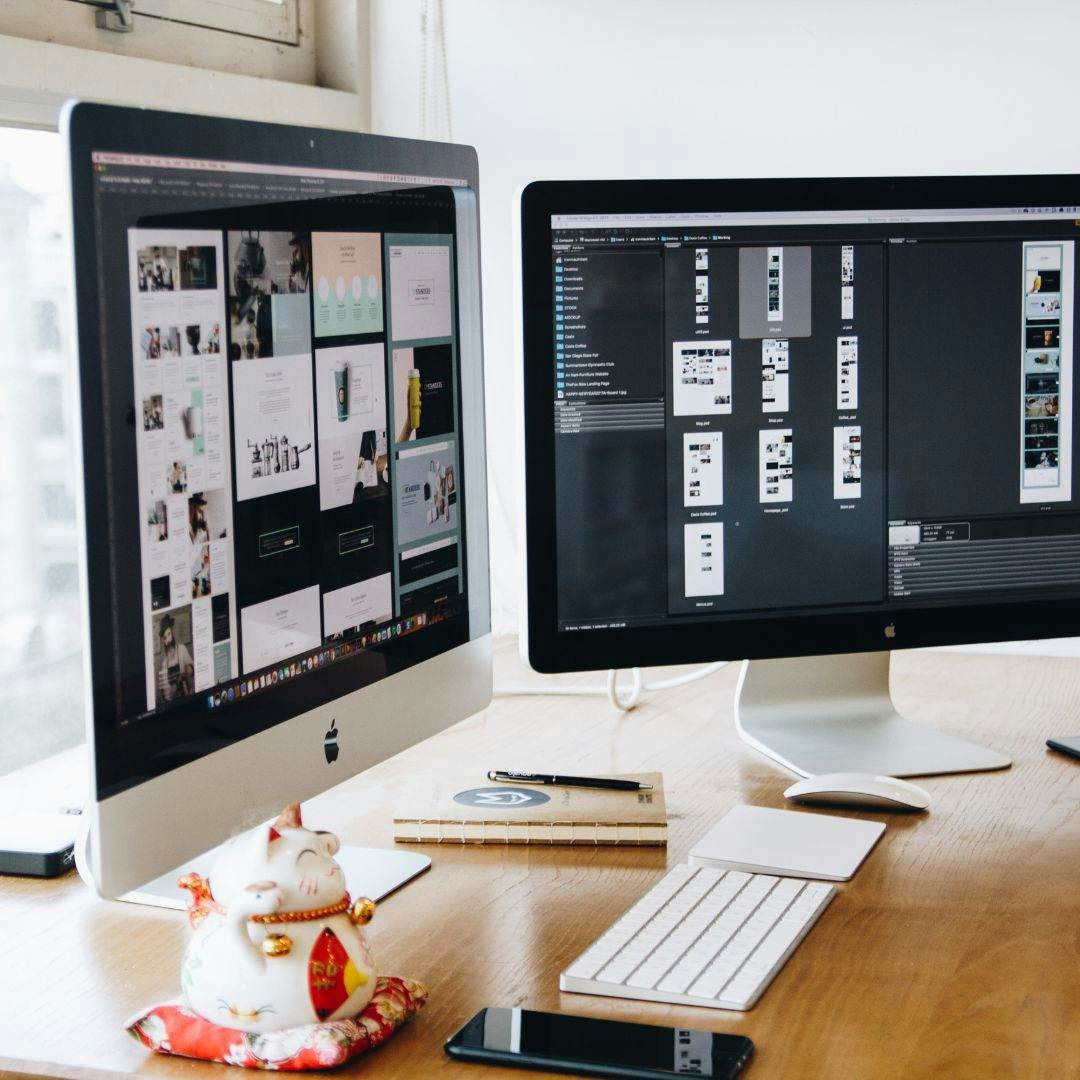
1. Introduction to Laravel Interviews
Laravel interviews are a crucial step for developers seeking to showcase their expertise in one of the most popular PHP frameworks available today. Understanding what to expect and how to prepare for these interviews can set you apart from other candidates. Laravel interviews often cover a wide range of topics, from basic PHP and Laravel knowledge to more complex architectural discussions.
A successful candidate will demonstrate proficiency in web development principles and a deep understanding of Laravel’s features and capabilities. Recruiters typically look for individuals who not only have the technical know-how but also can think critically and solve problems efficiently using Laravel’s tools and libraries.
To excel in a Laravel interview, you should be prepared to discuss your past projects and experiences with the framework. Knowing the ins and outs of Laravel’s MVC architecture, Eloquent ORM, Blade templating engine, and the Artisan command-line tool is essential. Furthermore, you’ll need to be conversant with Laravel’s approach to routing, middleware, security, and testing.
Having a solid grasp of database migrations, seeders, and managing dependencies with Composer will also be beneficial. Interviewers may also assess your understanding of performance optimization techniques specific to Laravel, as well as your experience integrating packages and extensions.
As you prepare, remember that Laravel interviews are not just about technical knowledge; they also evaluate your problem-solving strategies, your ability to work within a team, and your overall approach to coding. It’s important to communicate clearly and confidently, showing that you can contribute positively to the development process.
By familiarizing yourself with common interview questions and Laravel’s documentation, you can approach your interview with the confidence that comes from thorough preparation. Stay updated with the latest Laravel features and community best practices to ensure you present yourself as a knowledgeable and resourceful candidate.
2. Understanding the Laravel Framework Basics
Grasping the fundamentals of the Laravel framework is the foundation for any developer aiming to succeed in a Laravel interview. Laravel, an open-source PHP framework, is designed for the development of web applications following the Model-View-Controller (MVC) architectural pattern.
The first thing to understand about Laravel is its elegant syntax that aims to make the development process more enjoyable for the developer without sacrificing application functionality. It provides a rich set of features that are easy to understand and implement, and these features are what you need to be familiar with to excel in your interview.
Here are some of the key concepts of Laravel that you should be comfortable with:
- Routing: Know how Laravel handles routing and how you can define routes for your application.
- Middleware: Understand what middleware is and how it is used to filter HTTP requests entering your application.
- Controllers: Be able to explain the role of controllers and how they fit into the MVC architecture.
- Requests and Responses: Know how Laravel handles client requests and sends back responses.
- Views: Understand how views work within Laravel and how they are used to render the user interface.
- Blade Templating: Be familiar with Laravel’s Blade templating engine and how it can be used to create layouts and templates.
- Eloquent ORM: Know how the Eloquent ORM works for database interactions and the advantages it offers.
- Database Migrations: Understand the purpose of migrations and how they allow you to define your database schema.
- Validation: Be able to discuss Laravel’s built-in validation features and how you can apply them to data.
- Error and Exception Handling: Know how Laravel handles errors and exceptions, and the tools it provides for debugging.
- Automated Testing: Have an understanding of how automated testing is performed in Laravel.
- Service Container: Be aware of the service container and its role in managing class dependencies and performing dependency injection.
It’s crucial to have hands-on experience with Laravel, as practical knowledge is often more valuable than theoretical understanding. Make sure you have worked on Laravel projects and can discuss the specifics of how you implemented these basics.
Interviewers may ask questions that require you to illustrate how you’ve applied these concepts in real-world scenarios, so be prepared with examples from your experience. Knowing these basics will not only help you answer technical questions but also demonstrate to your interviewer that you have a solid understanding of the framework’s core components.
Stay current with Laravel’s official documentation, as it is an excellent resource for both learning and keeping up to date with the framework’s best practices and conventions. Being well-versed in the Laravel framework basics will show your potential employer that you have the foundational knowledge necessary to contribute to their projects and grow as a Laravel developer.
3. Advanced Laravel Concepts to Master
Mastering advanced Laravel concepts is essential for developers looking to distinguish themselves in a competitive job market. Beyond the basics, Laravel offers a range of advanced features and functionalities that allow for more efficient and scalable application development.
Understanding and utilizing service providers and facades is key for advanced Laravel development. Service providers are the central place for all Laravel application bootstrapping, including registering services, event listeners, and middleware. Facades provide a static interface to classes that are available in the application’s service container, making them easier to use without sacrificing testability and flexibility.
Advanced Eloquent relationships and querying techniques are another area to focus on. Laravel’s Eloquent ORM supports a variety of complex relationships such as polymorphic and many-to-many relationships. Knowing how to leverage these can greatly simplify database operations. Moreover, understanding how to use scopes, mutators, and accessors within Eloquent models will allow for more elegant manipulation of model data.
Event-driven programming in Laravel is another concept that can elevate your application’s functionality. Events in Laravel provide a simple observer implementation, allowing you to subscribe and listen for various events that occur within your application. Coupled with listeners, events can help you to decouple various parts of your application logic and make your code more maintainable.
Queues and job handling in Laravel are crucial for deferring the processing of a time-consuming task, such as sending emails, until a later time, which improves web application responsiveness. Understanding how to work with Laravel’s queue system can help you handle tasks asynchronously and provide a better user experience.
Advanced caching strategies can significantly improve the performance of your Laravel applications. Laravel supports popular caching backends like Memcached and Redis out of the box. Knowing how to effectively leverage caching can reduce the load on your database and speed up requests to your application.
Package development is a valuable skill in Laravel. Creating your own packages can allow you to modularize your code and share it across projects or with the Laravel community.
API resources and responses are critical for developers working with Laravel to build RESTful APIs. API resources allow you to transform your models and model collections into JSON effortlessly.
Here are some other advanced Laravel concepts to master:
- Task Scheduling: Understand how to use Laravel’s scheduler to execute scheduled tasks.
- Broadcasting: Learn how to implement real-time data broadcasting using websockets and Laravel Echo.
- Testing with Dusk and PHPUnit: Be proficient with browser testing using Laravel Dusk and PHPUnit for feature and unit testing.
- Laravel Passport for API Authentication: Know how to implement OAuth2 secured APIs using Laravel Passport.
It’s important to practice these advanced concepts by building applications or contributing to open-source projects. This will not only solidify your understanding but also provide concrete examples to discuss during interviews.
Interviewers may challenge you with scenarios that require advanced solutions, so be prepared to think critically and demonstrate your expertise. By mastering these advanced Laravel concepts, you will be well-equipped to tackle complex problems and stand out as a Laravel expert.
4. Familiarizing Yourself with MVC Architecture
Understanding the Model-View-Controller (MVC) architecture is fundamental when working with Laravel, as it is the framework’s core organizational pattern. MVC is an architectural pattern that separates an application into three main logical components: the model, the view, and the controller. Each of these components handles specific development aspects of an application.
The Model represents the application’s data structure, usually interacting with the database. It’s responsible for data retrieval, storage, and validation. In Laravel, models are mostly used in conjunction with Eloquent ORM, which provides an elegant and simple ActiveRecord implementation for working with your database. Each database table has a corresponding “Model” which is used to interact with that table.
The View is responsible for rendering the user interface of the application. It displays the data provided by the model in a format suitable for interaction, typically in the form of HTML. Laravel utilizes the Blade templating engine, which is powerful yet simple to use, allowing developers to create dynamic content with minimal effort.
The Controller acts as an intermediary between models and views. It processes incoming requests, manipulates data through the model, and renders the final view to the user’s browser. Controllers in Laravel are used to group related request handling logic within a single class.
Laravel’s MVC pattern promotes clean separation of concerns, which means:
- Your model code is separate from your user interface code, which makes the codebase easier to manage and test.
- The controller decides what data to pull from the model and which view to display, based on the user’s request and other factors.
To familiarize yourself with Laravel’s MVC architecture, you should:
- Dive into the Laravel documentation to understand how routing works, how controllers are created, and how views are rendered.
- Create controllers using the
artisan make:controller
command and define methods for handling various HTTP requests within those controllers. - Explore how to pass data to views and how to build dynamic content with Blade templating.
- Learn how to define Eloquent models and use them to interact with your database through various relationships and methods.
By using this architecture, Laravel ensures clarity in logic and presentation, which is especially beneficial in collaborative environments where clear and readable code is essential. Practical experience with MVC in Laravel will greatly enhance your understanding and ability to discuss these concepts during an interview.
To demonstrate your grasp of MVC during an interview, explain how you have used MVC in previous projects and the benefits it brought to your workflow. Discuss any challenges you faced and how you overcame them, using specific examples. This will not only show that you understand MVC conceptually but also that you can effectively put it into practice in real-world scenarios.
5. Eloquent ORM: Best Practices and Usage
Eloquent ORM is Laravel’s built-in Object-Relational Mapping tool and is widely admired for its simplicity, elegance, and readability. It is crucial to follow best practices to make the most of Eloquent’s capabilities and ensure your application remains performant and maintainable.
Make use of Eloquent’s relationships efficiently to simplify data operations. Laravel supports several types of relationships such as one-to-one, one-to-many, many-to-many, and polymorphic relationships. Defining these relationships correctly in your Eloquent models will allow you to perform complex data queries with ease.
Avoid the N+1 query problem by eager loading relationships. This occurs when you query a model and then run a separate query for each related model. Using methods like with()
to preload relationships can dramatically reduce the number of queries that run against your database.
Use scopes for common query constraints. Local scopes allow you to define common sets of query constraints that you can easily reuse throughout your application. This helps in keeping your database queries readable and maintainable.
Leverage accessors and mutators to format Eloquent attribute values when you retrieve or set them on model instances. Accessors and mutators provide a way to alter data before it’s saved to the database or after it’s fetched from the database.
Take advantage of mass assignment protection. Always define fillable or guarded properties on your models to protect against mass-assignment vulnerabilities. This ensures only the specified attributes are affected when passing an array to the model’s create or update methods.
Use Eloquent collections to work with multiple models. Eloquent returns results as Collection objects, which offer a suite of powerful methods for traversing, filtering, and mapping over the items.
Keep Eloquent queries readable. Break down complex queries into smaller, chainable methods to improve readability and make debugging easier.
Stay aware of the underlying database. While Eloquent abstracts much of the database interaction, having a good understanding of SQL and the database you are working with can help you write more efficient models and queries.
Don’t overuse Eloquent in complex queries. Sometimes it’s more efficient to write a raw query or use the query builder instead of forcing everything through Eloquent.
When dealing with large datasets, make sure to use chunking. The chunk()
method retrieves a small chunk of the results at a time and feeds each chunk into a closure for processing. This method is particularly useful for writing Artisan commands that process thousands of records.
Regularly review your Eloquent usage for potential optimizations, especially after Laravel updates, as new features and improvements can affect best practices.
By following these best practices with Eloquent ORM, you will ensure that your Laravel application takes full advantage of this powerful and expressive ORM for efficient, elegant database manipulation. Remember, the best practices are not only about writing efficient code but also about writing code that is easy to read, maintain, and scale as your application grows.
6. Artisan Commands You Should Know
Laravel’s Artisan command-line interface provides a number of helpful commands that are essential for Laravel development. Familiarizing yourself with these commands can greatly streamline your development process and enhance your productivity.
Key Artisan commands include:
php artisan list
: Displays a list of all available Artisan commands.php artisan help [command_name]
: Provides detailed information about a specific command, including its arguments and options.php artisan make:[type] [name]
: A set of generators that quickly scaffold various types of classes, like controllers, models, and migrations.php artisan migrate
: Runs the database migrations. It’s often followed byphp artisan migrate:rollback
to undo the last database migration, orphp artisan migrate:reset
to roll back all migrations.php artisan db:seed
: Seeds the database with test data from your seed classes.php artisan tinker
: Allows you to interact with your entire Laravel application on the command line, including Eloquent ORM, tasks, and more.php artisan cache:clear
: Clears the application cache.php artisan config:cache
: Caches the configuration files, which is useful in production for performance optimization.php artisan route:list
: Shows a list of all registered routes, including their URIs, names, and associated actions.php artisan view:clear
: Clears all compiled Blade templates.php artisan storage:link
: Creates a symbolic link frompublic/storage
tostorage/app/public
for public access to files.
Remember to use migrations and seeders for managing your database schema and initial data. Commands like php artisan make:migration
and php artisan make:seeder
are invaluable for creating the necessary classes.
For maintenance and troubleshooting, commands such as php artisan down
and php artisan up
are used to put the application into and out of maintenance mode, respectively.
Take advantage of Artisan’s capabilities to optimize your application. Utilize commands like php artisan optimize
to compile commonly used classes into a single file for faster loading.
Creating custom Artisan commands can also be extremely powerful. If you find yourself repeatedly performing complex tasks, consider writing a custom command using php artisan make:command
. This can encapsulate functionality and make it easily reusable.
To excel in a Laravel interview, being able to discuss and demonstrate your use of Artisan commands is a strong indicator of your practical experience with the framework. Describe scenarios where you have used Artisan commands to solve problems or automate tasks during your development workflow.
Practice using these commands regularly to ensure that you are comfortable with them during development and can speak about them confidently in an interview setting. Artisan is a powerful tool that, when used effectively, can significantly enhance your Laravel development experience.
7. Laravel Security Features and Best Practices
Laravel is well-regarded for its robust security features, designed to protect web applications from common security vulnerabilities. Understanding and implementing these features and best practices is crucial to maintain the integrity of your applications.
Employ Laravel’s built-in user authentication for a secure and straightforward implementation. The auth
scaffolding provides a full authentication system for login, registration, and password reset out of the box.
Use authorization techniques such as Gates and Policies to control access to resources within your application. These allow you to define clear logic for who can perform actions on resources.
Be mindful of Cross-Site Request Forgery (CSRF) attacks. Laravel automatically generates a CSRF token for each active user session managed by the application. This token is used to verify that the authenticated user is the one actually making the requests to the application.
Protect against SQL injection attacks with Eloquent ORM or the query builder. Both use PDO parameter binding to prevent this type of vulnerability.
Guard against Cross-Site Scripting (XSS) by escaping data output. Use Blade’s {{ }}
syntax which will automatically escape any output to be safe for rendering in HTML.
Utilize HTTPS to prevent man-in-the-middle (MITM) attacks. Configure your application to always use a secure HTTPS connection by setting the APP_URL
in your .env
file to an HTTPS address and using the secure_asset()
and secure_url()
helpers.
Store passwords securely using Laravel’s hashing functionality. Laravel uses the Bcrypt hashing algorithm for encrypting passwords, which is a secure way of storing passwords in your database.
Limit the number of authentication attempts to prevent brute force attacks with Laravel’s built-in rate limiting features.
Keep your Laravel application up to date to ensure you have the latest security fixes. Regularly check for updates and apply them to your application.
Regularly audit your code and dependencies for security vulnerabilities. Use tools like Laravel’s built-in php artisan security:check
command or third-party services to check your application’s dependencies for known security vulnerabilities.
Implement proper error handling to avoid exposing sensitive application details. Configure your logging settings and exception handling to prevent sensitive data leakage in error messages.
By adhering to these security practices, you can leverage Laravel’s features to build secure applications and demonstrate your commitment to security during your interview. Discussing specific instances where you applied these security measures can serve as a testament to your skills and awareness regarding application security.
8. Performance Optimization in Laravel
Performance optimization in Laravel is a critical aspect of developing efficient and responsive applications. By implementing best practices for performance, you can ensure that your Laravel application runs smoothly and can handle a high volume of traffic.
Utilize caching to improve response times. Laravel supports different caching backends like Memcached and Redis. You should cache configurations, routes, views, and responses wherever possible to reduce load times.
Minimize the use of Eloquent ORM in complex queries. While Eloquent is powerful and developer-friendly, it can be slower than the query builder for very complex database operations. Use raw queries or the query builder when necessary to optimize performance.
Optimize database queries to reduce execution time. Regularly use tools like Laravel Debugbar to monitor and optimize your queries. Avoid N+1 problems by eager loading relationships, and use indexing and query caching to speed up database access.
Compile assets and use a content delivery network (CDN). Use Laravel Mix to compile, minify, and concatenate your CSS and JavaScript files. Delivering assets through a CDN can also greatly reduce latency and improve loading times for your users.
Leverage lazy loading for service providers. Mark your service providers as deferred if they are not needed on every request, which can cut down on bootstrapping time.
Take advantage of model events for efficient data handling. Model events allow you to hook into various points of a model’s lifecycle, such as when it’s being created, updated, or deleted. This can help you avoid unnecessary processing.
Utilize queueing systems for time-consuming tasks. By pushing tasks such as sending emails or generating reports to a queue, you can free up your application to handle more web requests, thus improving overall responsiveness.
Implement HTTP/2 to reduce loading times. HTTP/2 offers performance improvements over HTTP/1.1, including reduced latency and improved loading times for web pages.
Use pagination to handle large datasets. Instead of loading thousands of records at once, use Laravel’s pagination to load a small subset of data at a time.
Profile and monitor your application regularly. Keep track of your application’s performance using profiling tools and Laravel’s built-in logging capabilities. This will help you identify and address bottlenecks promptly.
Optimize your environment configuration. Ensure that your server is configured correctly for running Laravel applications. For instance, using PHP OPcache can significantly increase PHP’s performance by storing precompiled script bytecode.
Regularly update your Laravel version to benefit from the latest performance improvements. New releases often include optimizations that can make your application faster.
By following these performance optimization techniques in Laravel, you can create a seamless experience for your users and maintain a robust application. During an interview, being able to discuss specific instances where you have successfully optimized a Laravel application can demonstrate your technical proficiency and understanding of efficient application design.
9. Testing Your Laravel Application
Thorough testing is a cornerstone of reliable and maintainable software development, and Laravel provides a suite of tools to help you test your application effectively. Laravel is built with testing in mind, and it’s essential to understand how to use these tools to create a robust test suite for your Laravel applications.
Utilize PHPUnit for both unit and feature tests. Laravel is configured to work with PHPUnit out of the box, and a phpunit.xml
file is already set up for your application. Unit tests focus on a small portion of code, like a single method in a class, while feature tests examine the interaction between various parts of the application, such as a series of HTTP requests.
Leverage Laravel’s built-in testing helpers and assertions to simplify the process of testing your application. These include methods for making requests, interacting with the session, handling cookies, and checking for specific responses.
Implement browser tests with Laravel Dusk if your application has complex JavaScript interactions. Dusk provides an expressive API for browser automation and testing, allowing you to perform user actions and assert that visible text or elements are present on the page.
Mock external services and APIs to test your application in isolation. Use Laravel’s built-in facades and helper methods to mock calls to external services, ensuring your tests are not dependent on third-party services.
Practice Test-Driven Development (TDD) where appropriate. With TDD, you write tests before you write the corresponding code, which can lead to more thoughtful design decisions and a more testable codebase.
Use database migrations and factories to set up your test database environment. Laravel’s migrations and seeders allow you to create a consistent database structure for your tests, while factories can generate test data quickly and easily.
Test different layers of your application separately. Ensure that you have unit tests for your models, controllers, and middleware. This helps to catch issues at the layer where they occur and simplifies debugging.
Take advantage of Laravel’s environment and configuration handling for testing. You can set up different environment configurations for testing to ensure that your tests do not interfere with your production or development environments.
Regularly run your entire test suite. As part of your development workflow, make it a habit to run tests before pushing code changes to catch any regressions early.
Integrate continuous integration (CI) services to automatically run your tests on various environments whenever you push new code updates. This helps to ensure that your application works as expected across different configurations and dependencies.
By following these practices and making testing an integral part of your development process, you can build confidence in your Laravel application’s functionality and integrity. In an interview setting, discussing how you’ve implemented testing strategies can showcase your commitment to quality and your proactive approach to application development.
10. Managing Dependencies with Composer
Composer is a crucial tool for managing dependencies in PHP applications, including those built with Laravel. It handles the installation and updating of packages that your project relies on, ensuring that you have the specific versions needed and that they are compatible with each other.
Understand the composer.json
file in your Laravel project. This file declares the libraries your project depends on and defines constraints to ensure compatibility. It also includes autoload settings for your application’s classes.
Regularly use composer update
to keep your packages up-to-date. This will update your composer.lock
file with the latest compatible versions of your dependencies. However, be cautious with this command in production environments, as it may install non-backward compatible updates. For production, it’s often safer to use composer install
, which installs the exact versions listed in your composer.lock
file.
Leverage version constraints wisely in your composer.json
. Using semantic versioning constraints (such as ^1.2
or ~1.2.3
) allows Composer to install updates that are backward compatible. Avoid using dev-master
as a version constraint, as this can lead to unstable versions being installed.
Use composer require
to add new packages to your project. This command updates both your composer.json
and composer.lock
files and installs the package.
Understand the difference between “require” and “require-dev” in composer.json
. Packages listed under “require” are necessary for your application to run, while “require-dev” packages are only needed for development purposes, like testing tools or code generators.
Utilize the autoload
feature to optimize the autoloading of classes. Composer provides PSR-4 autoloading, which maps namespaces to directories. You can also use the composer dump-autoload
command to regenerate the autoload files if you add new classes manually.
Manage package stability with minimum-stability and prefer-stable settings. These settings allow you to specify the stability level for your packages, ranging from stable to dev.
Use Composer scripts to automate common tasks. Define scripts in your composer.json
to run tasks like tests, cache clearing, or database migrations with Composer’s event hooks.
Be cautious with third-party packages. Always review the code quality, maintainability, and licensing of packages before including them in your project. Also, consider the package’s update frequency and community support.
Incorporate Composer into your deployment process. Ensure that you have a strategy for running composer install
as part of your deployment to install the correct versions of your dependencies on production servers.
By mastering Composer for dependency management in Laravel, you demonstrate to interviewers that you have a solid grasp on maintaining a stable and efficient development environment. Discussing how you’ve managed dependencies in past Laravel projects can highlight your technical skills and your ability to keep a Laravel application up-to-date and secure.
11. Database Migrations and Seeders in Laravel
Database migrations in Laravel provide an easy and consistent way to modify your database schema over time. They are like version control for your database, allowing a team to define and share the application’s database schema definition. Migrations typically use a simple PHP syntax and can be rolled back if necessary, permitting changes to be applied and reverted in a controlled manner.
To create a new migration, you can use the php artisan make:migration
command followed by a descriptive name. This will create a new migration file in the database/migrations
directory. Within this file, you will define the changes to the database tables, such as creating or modifying columns.
When you run the php artisan migrate
command, Laravel will execute the outstanding migrations. It’s important to ensure that migrations are run in the correct order, and Laravel handles this by using the migration filename, which includes a timestamp.
Seeders in Laravel are used to populate your database with initial data. They are useful for testing and setting up initial states for your application’s database. You can create a new seeder using php artisan make:seeder
and include the command php artisan db:seed
to run it.
Use the --seed
flag in conjunction with the migrate
command to run your seeders immediately after migrating. The php artisan migrate:refresh --seed
command is particularly useful during development as it allows you to reset your database and re-run all of your migrations along with the database seeders.
Laravel provides a powerful query builder and Eloquent ORM for interacting with your database. However, it is often necessary to have a raw SQL option. Migrations support this through the DB::statement
method, allowing execution of arbitrary SQL commands.
Always test your migrations and seeders thoroughly to prevent issues in production. It’s also good practice to use Laravel’s multiple database connections feature to test migrations and seeders on a separate database connection.
Remember to version control your migration and seeder files. They are part of your application’s codebase and should be included in your version control system, ensuring that any team member can deploy the application and set up the database.
By effectively utilizing migrations and seeders, you can maintain a clear and consistent database schema history, automate the deployment of schema changes, and ensure that your development, staging, and production environments are synchronized. Being able to discuss and demonstrate your experience with database migrations and seeders will be beneficial during a Laravel interview. It shows your understanding of database version control and how to manage data throughout the lifecycle of a Laravel application.
12. Blade Templating Engine Essentials
The Blade templating engine is an essential feature of Laravel, providing a powerful yet simple way to work with HTML templates. With Blade, you can create dynamic web pages that incorporate PHP code easily and cleanly.
Blade templates are defined in .blade.php
files and typically stored in the resources/views
directory. These templates allow you to define sections, extend layouts, and include subviews, which help maintain a DRY (Don’t Repeat Yourself) principle in your views.
Utilize Blade’s template inheritance capabilities. By defining a master layout with @yield
directives, you can specify where child templates should inject content. Child templates can then @extend
the master layout and @section
to fill in the content.
Make use of Blade’s components and slots for reusable code. Components can be thought of as custom HTML elements that you can pass data into, making them highly reusable and testable. Slots allow you to insert content into different parts of the component.
Blade’s control structures are key for dynamic content. Use @if
, @foreach
, @for
, and other directives to control what gets rendered based on the data passed to the view. Blade’s syntax is cleaner and more intuitive than using plain PHP in your templates.
Take advantage of Blade’s template compilation. Blade views are compiled into plain PHP code and cached until they are modified, which means Blade adds essentially zero overhead to your application.
Escape output to prevent Cross-Site Scripting (XSS) attacks. By default, Blade {{ }}
statements are automatically sent through PHP’s htmlspecialchars
function to prevent XSS. Always use the escaped output unless you are sure the output is safe to render as HTML.
Leverage Blade’s service injection to access Laravel’s services directly within templates. Using the @inject
directive, you can retrieve services from the container, which is particularly useful for reading configuration values or using services without passing them from controllers.
Optimize your Blade views by flattening deeply nested views and reducing computations. Too many nested views can affect performance, and complex computations should be done in the controller or model, not in the view.
Customize Blade’s behavior with custom directives if the built-in ones don’t meet your needs. You can define your own Blade directives using the directive
method provided by the Blade facade.
Remember to use comments in your Blade templates for clarity. Blade comments with {{-- --}}
will not be included in the HTML returned to the browser, keeping your markup clean.
Understanding and applying these Blade templating engine essentials will enable you to build responsive, dynamic, and secure web applications with Laravel. During a Laravel interview, demonstrating your knowledge of Blade’s features and best practices can illustrate your proficiency in creating well-structured and maintainable views.
13. Understanding Laravel Middleware
Middleware in Laravel serves as a powerful way to filter HTTP requests entering your application. It operates between the request and response, allowing you to inspect and even modify the request and response objects.
Middleware can be used for a variety of tasks, such as authentication, logging, and CORS. For example, the built-in auth
middleware can be used to ensure that a user is logged in before allowing access to certain routes.
You can create your own middleware by using the php artisan make:middleware
command. This will generate a new middleware class in the app/Http/Middleware
directory. Within this class, you have the ability to handle an incoming request, perform actions, and then either pass the request further down the application or return a response.
Middleware can be registered globally, assigned to specific routes, or grouped within route groups. Global middleware will run on every HTTP request to the application, while route middleware can be applied to individual routes as needed.
Understand how to pass additional parameters to route middleware. This is useful when you need to provide dynamic conditions for middleware, such as specifying a particular role or permission.
Remember to use middleware parameters sparingly to keep your middleware flexible and reusable. Parameters can make your middleware more complex, so they should be used only when necessary.
Chain multiple middleware for more granular control over your requests. By combining different middleware, you can create a layered security and filtering mechanism that’s tailored to your application’s needs.
Take advantage of the Terminate
middleware feature in Laravel. This allows your middleware to perform tasks after the HTTP response has been sent to the browser, which is useful for performing tasks that do not need to be completed before the response is sent, like logging or sending emails.
Be mindful of the order in which middleware is executed. The order of global middleware is defined in your application’s HTTP kernel, and the order can affect the outcome of your requests and responses.
Utilize middleware for API rate limiting to protect your application from abuse. Laravel’s built-in ThrottleRequests
middleware can be applied to API routes to limit the number of requests a user can make within a certain time frame.
Understanding and leveraging middleware correctly can greatly enhance the functionality and security of your Laravel application. Discussing specific middleware you’ve implemented and the reasoning behind it can provide valuable insights into your problem-solving abilities during a Laravel interview.
14. Laravel Packages and Extensions
Laravel’s ecosystem is enriched by a wide array of packages and extensions, which extend the framework’s core functionality and provide convenient ways to add features to your applications. Knowing how to integrate and utilize these packages and extensions is a valuable skill for any Laravel developer.
Popular packages such as Laravel Passport, Laravel Socialite, and Laravel Cashier offer robust solutions for common tasks like API authentication, social media integration, and subscription billing management. These packages are maintained by the Laravel team, ensuring high-quality and compatibility with the framework.
Choose packages wisely based on their quality, documentation, and maintenance. Favor packages that are well-documented, actively maintained, and have good community support. Check repositories on GitHub for activity and issues to gauge the health of a package.
Understand how to use Composer to install and manage third-party packages. Adding a package is typically as simple as running composer require package/name
from your terminal, which will download the package and update your composer.json
and composer.lock
files.
Familiarize yourself with Laravel’s service providers, which are the connection point between your Laravel application and a package. Service providers bind package functionality into the service container, register routes, and perform other bootstrapping functions.
Use package discovery to automatically register service providers and aliases. Laravel’s package discovery feature automatically detects and registers service providers and facades from your project’s dependencies, simplifying the setup process.
When needed, publish a package’s configuration files using the php artisan vendor:publish
command. This allows you to customize the settings of the package without altering the package’s core files, making it easier to update the package in the future.
Consider creating your own Laravel packages for reusable code. If you find yourself using the same class or functionality across multiple projects, packaging that code can save time and help maintain consistency.
Be aware of the potential security risks when using third-party packages. Always review the source code and ensure you trust the package’s authors before including it in your project. Keep your dependencies up to date to incorporate security patches and updates.
Test the integration of packages thoroughly. Ensure that the package works well with your application and does not introduce any unexpected behaviors or conflicts with other packages or services.
During a Laravel interview, discussing your experience with integrating and managing packages can illustrate your ability to enhance applications efficiently. Being able to explain the process of selecting a package, integrating it into a Laravel application, and overcoming any challenges faced will demonstrate your competence and familiarity with the Laravel ecosystem.
15. Preparing for Behavioral Interview Questions
Preparing for behavioral interview questions is just as important as technical preparation when acing a Laravel interview. Behavioral questions aim to assess your soft skills, such as teamwork, communication, leadership, and problem-solving abilities. These questions often begin with phrases like “Tell me about a time when…” or “Give me an example of…”
Reflect on your past experiences and have concrete examples ready. Think about different situations you’ve encountered in your career where you had to overcome challenges, work with difficult team members, lead a project, or adapt to change. Structure your responses using the STAR method (Situation, Task, Action, Result) to provide a concise and compelling narrative.
Demonstrate effective communication skills. Practice explaining your thought process clearly and concisely. Good communication is not only about speaking well but also about active listening and being able to understand and address the interviewer’s questions appropriately.
Highlight your teamwork and collaboration skills. Be prepared to discuss how you have contributed to a team environment, managed conflicts, and supported your colleagues. Emphasize the importance of diverse perspectives and the value of working towards a common goal.
Show your problem-solving abilities by discussing how you approach complex issues. Explain the steps you take to analyze a problem, explore different solutions, and implement the most effective one. Be prepared to talk about a specific technical problem you solved and how it benefited the project or the team.
Exhibit adaptability and a willingness to learn. Share examples of how you’ve embraced new technologies or methodologies, adapted to changing project requirements, or continued to grow your skills in the face of challenges.
Discuss your time management and organizational skills. Describe how you prioritize tasks, handle deadlines, and stay productive under pressure. Providing examples of successful project deliveries or how you managed multiple responsibilities can be particularly effective.
Explain your motivation and passion for development, particularly within the Laravel framework. Talk about what drives you, why you enjoy working with Laravel, and how you stay updated with the latest trends and best practices in the development community.
Be authentic and let your personality shine through. Employers are looking for candidates who not only have the technical skills but also fit well within their company culture. Being genuine and personable can make a lasting impression.
Remember to ask questions at the end of the interview. This shows your interest in the role and the company, and can also help you determine if the job is the right fit for you.
By preparing for behavioral interview questions, you demonstrate to potential employers that you possess the soft skills necessary to be a valuable team member and contribute positively to the company’s goals and culture. Discussing real-life examples that highlight your strengths and learning experiences will make a strong impact during your Laravel interview.
16. Laravel Interview Common Questions and Answers
Expect to face a range of common questions during a Laravel interview, which may touch upon both technical aspects of the framework and general programming concepts. The following are some frequently asked questions along with sample answers to help you prepare:
- What is Laravel and what makes it different from other PHP frameworks?
Laravel is a modern, open-source PHP framework designed for web application development following the MVC pattern. What sets Laravel apart is its elegant syntax, robust features like Eloquent ORM, built-in authentication, and extensive community support. It also emphasizes convention over configuration, which streamlines development by reducing the need for repetitive coding tasks.
How does the MVC architecture work in Laravel?
In Laravel, the Model represents the data and business logic, the View is responsible for presenting data to the user, and the Controller handles user input and transforms it into a response. Laravel’s MVC architecture helps in organizing code, promoting a separation of concerns, and making the application easier to maintain and scale.
Can you explain route middleware in Laravel?
Route middleware in Laravel is used to filter HTTP requests entering the application. It can be applied to individual routes or groups of routes to handle tasks like authentication and authorization, logging, or modifying the request or response objects. Middleware allows for the encapsulation of common pre- and post-request logic.
What are Eloquent relationships and give an example?
Eloquent relationships are methods defined in Eloquent models that allow you to set up associations between different models. For example, a
User
model might have aposts
method that returns the result of$this->hasMany(Post::class)
, defining a one-to-many relationship between users and posts.How do you prevent SQL injection in Laravel?
Laravel uses PDO parameter binding to protect against SQL injection attacks. This means all queries built using Eloquent ORM or Laravel’s query builder are protected by default. Developers should avoid using raw queries; if necessary, they should use parameter binding to prevent SQL injection.
What is database migration and why is it important?
Database migrations are a way of version controlling database schemas and sharing them among team members. They allow developers to define changes to the database structure using PHP code. Migrations are important because they allow for easy modification, rollback, and deployment of database schemas.
How does Laravel handle testing?
Laravel is built with testing in mind, providing support for PHPUnit out of the box. It includes a variety of testing utilities and assertions to streamline the process of writing tests, covering unit tests, feature tests, and browser tests with Laravel Dusk.
What are service providers in Laravel?
Service providers in Laravel are the central place for all of the application’s bootstrapping. They tell Laravel about the services the application provides, such as binding classes into the service container, registering routes, and setting up event listeners.
How do you update a Composer package in Laravel?
To update a Composer package, you can run
composer update vendor/package
to update a specific package or simplycomposer update
to update all packages. It’s important to review the changes and test the application after updating to ensure compatibility.What is the purpose of Laravel’s
artisan
command?- Laravel’s
artisan
is a command-line interface that provides a number of helpful commands to assist with Laravel application development, such as generating boilerplate code, managing database migrations, and running tests. It’s a powerful tool for automating repetitive tasks.
- Laravel’s
Being well-prepared with answers to these common questions will showcase your technical knowledge and experience with Laravel, helping you to stand out in an interview. Remember to tailor your answers based on your own experiences and understanding of the framework.
17. Tips for Project Portfolio Showcase
A well-curated project portfolio can significantly enhance your chances during a Laravel interview. It offers tangible proof of your technical abilities and gives insight into your problem-solving skills. Here are some tips to effectively showcase your projects:
Select projects that highlight your proficiency with Laravel. Choose a variety of projects that demonstrate your ability to work with different aspects of the framework, from routing and middleware to Eloquent ORM and Blade templating.
Ensure your code is clean, well-documented, and follows Laravel’s conventions. This reflects your attention to detail and understanding of best practices, which is crucial for collaborative projects.
Include projects that solve real-world problems. Employers are interested in how you apply your skills to practical situations. Describing the challenges you faced and how you overcame them using Laravel can be very persuasive.
Demonstrate your capability to write tests. Share examples where you have used PHPUnit or Laravel Dusk to write tests for your code. This shows that you value quality and stability in your development process.
Showcase your understanding of database design. Including projects that feature complex database relationships or efficient database queries can highlight your backend development skills.
Highlight any contributions to open-source projects or packages you’ve developed. This demonstrates your engagement with the community and willingness to share knowledge, as well as your ability to write reusable code.
Discuss performance optimization. Be prepared to explain any steps you took to optimize the performance of your Laravel applications, which can be a significant concern for potential employers.
If you’ve worked with modern front-end frameworks in conjunction with Laravel, showcase this. Projects that demonstrate your full-stack capabilities, including API usage, can set you apart.
Include a variety of project sizes. Displaying both large and small projects can illustrate your ability to handle different scales of development work.
Prepare a brief explanation for each project. Be ready to discuss the purpose of the project, your role, the Laravel features you used, and any challenges you encountered.
Ensure your portfolio is accessible and well-organized. A GitHub profile or personal website that clearly presents your projects, with READMEs and documentation, makes it easy for interviewers to review your work.
Be honest about collaborative projects. Clearly state your specific contributions if the project was a team effort.
Your project portfolio is your chance to make a strong impression on potential employers. It provides a platform to demonstrate your skills, creativity, and passion for Laravel development. Prepare to walk interviewers through your portfolio, discussing your thought process and the decisions you made along the way.
18. Following Up After the Interview
Following up after a Laravel interview is an important step in the job application process. It shows your continued interest in the position and can help keep you top of mind for the hiring manager. Here are some effective strategies for following up:
Send a thank-you email within 24 hours of the interview. Express your gratitude for the opportunity to interview and reiterate your enthusiasm for the role. This is not just polite but also professional and can differentiate you from other candidates.
Personalize your follow-up communications. Mention specific topics discussed during the interview that resonated with you or additional thoughts you have on a subject that was brought up. This demonstrates your attentiveness and engagement with the interview process.
Keep your follow-up message concise and to the point. While you want to express your interest and appreciation, respect the interviewer’s time by being direct and brief.
Use the follow-up as an opportunity to provide additional information. If there was a question you felt you could have answered better or if you have examples of your work that are relevant to the discussion, include them in your follow-up.
Reaffirm your fit for the position and the value you can bring to the team. Highlight your relevant skills and experiences that align with the job description and the company’s goals.
Be patient and professional. While you may be eager to hear back, understand that the hiring process can take time. It’s appropriate to ask about the timeline for a decision during the interview, and you can reference this in your follow-up.
If you haven’t heard back within the expected timeframe, it’s appropriate to send a polite inquiry. Reiterate your interest in the position and inquire if there has been an update on the hiring decision.
Prepare for any response. Whether you receive a job offer, are invited for another interview, or are informed that the position has been filled, respond graciously and professionally.
Following up after your interview demonstrates your professionalism and interest in the position. It can also provide an additional touchpoint to reinforce your suitability for the role. By handling the follow-up with courtesy and tact, you leave a positive impression regardless of the outcome.
19. Conclusion and Next Steps
The conclusion of your Laravel interview journey marks the beginning of the next phase in your career. Whether you receive an offer or are continuing your job search, it’s important to reflect on the experience and consider your next steps.
If you receive a job offer, carefully review the terms and conditions. Ensure that the role meets your career goals and expectations. If you have any questions or need clarification, don’t hesitate to reach out to the employer.
In case you’re not selected for the position, seek feedback from the interviewer. This can provide valuable insights into areas for improvement and help you prepare for future opportunities.
Continue to expand your knowledge and skills in Laravel. The tech industry is always evolving, and staying current with the latest Laravel features, best practices, and community contributions will enhance your expertise and employability.
Keep networking within the Laravel and PHP communities. Attend meetups, participate in forums, contribute to open-source projects, or write blog posts. These activities will increase your visibility and can lead to new opportunities.
Maintain a positive attitude and stay persistent in your job search. Each interview is a learning experience that brings you closer to finding the right position that matches your skills and aspirations.
Remember that the journey to becoming a successful Laravel developer is continuous. Embrace the challenges and celebrate the milestones along the way.