Software Services
For Companies
For Developers
Portfolio
Build With Us
Table of Contents:
Get Senior Engineers Straight To Your Inbox

Every month we send out our top new engineers in our network who are looking for work, be the first to get informed when top engineers become available

At Slashdev, we connect top-tier software engineers with innovative companies. Our network includes the most talented developers worldwide, carefully vetted to ensure exceptional quality and reliability.
Build With Us
How To Ace Your React Native Interview/
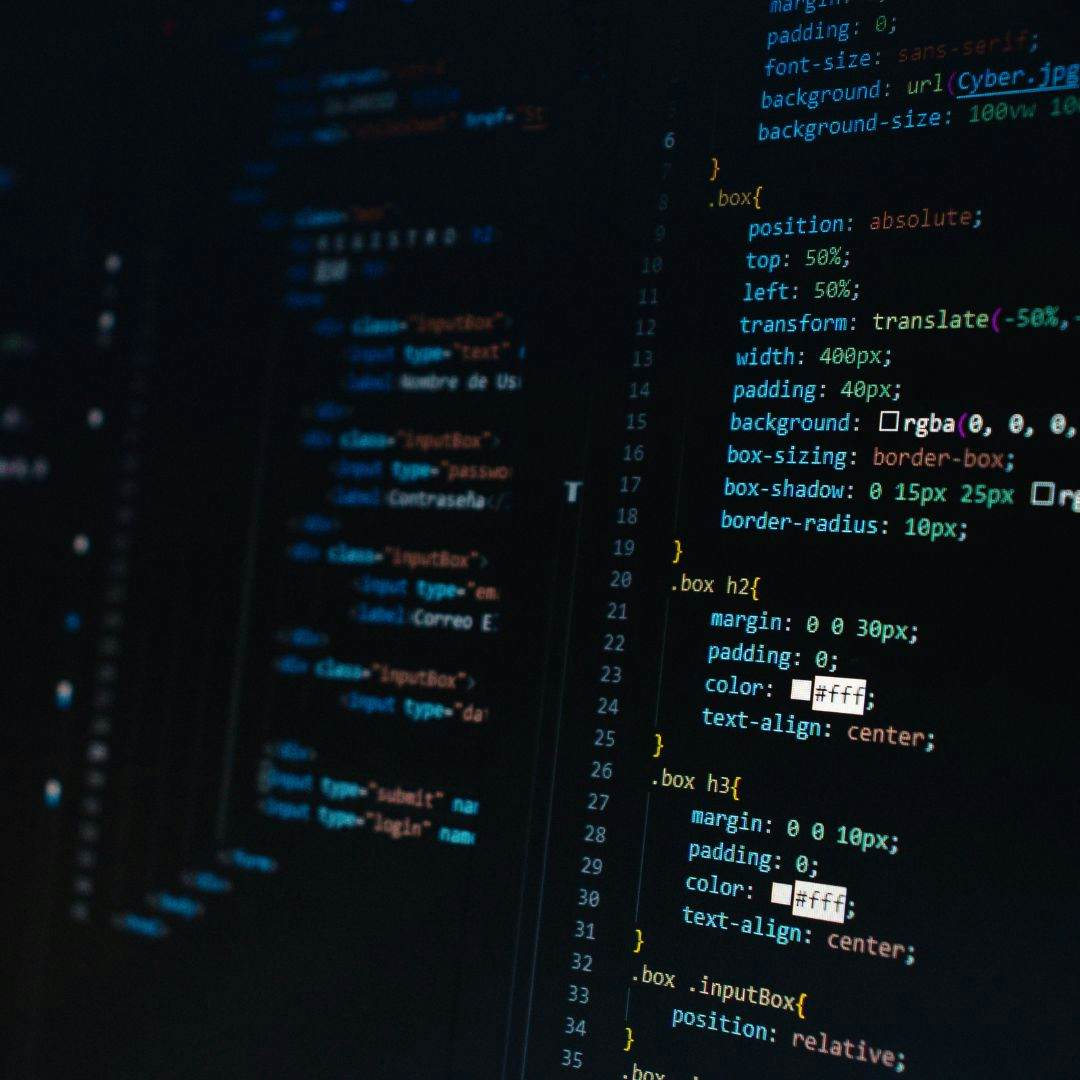
1. Introduction to React Native
React Native is an open-source framework developed by Facebook that enables developers to build mobile applications using JavaScript and React. It’s designed for creating natively-rendered apps for iOS and Android with a single codebase, which makes it a popular choice among developers who want to streamline their development process without sacrificing performance or user experience.
Getting started with React Native requires a good understanding of JavaScript and the fundamentals of React, such as JSX, components, state, and props. Moreover, it embraces the use of native components which allows for the creation of high-quality user interfaces that are indistinguishable from those built with native SDKs.
As React Native grows in popularity, the demand for developers with proficiency in this framework has been increasing. A strong grasp of React Native can open many doors for opportunities in mobile app development. Preparing for a React Native interview involves not only technical knowledge but also an understanding of best practices and the latest developments in the framework.
For those looking to ace their React Native interviews, it’s crucial to delve into the core concepts, familiarize oneself with commonly used libraries and tools, and stay up-to-date with the latest features and updates. It’s also important to demonstrate one’s practical skills through a well-crafted portfolio that showcases real-world React Native projects.
As you embark on your journey to master React Native and prepare for interviews, remember that a deep understanding of the framework, combined with the ability to apply it to solve complex problems, will set you apart as a strong candidate in the competitive landscape of mobile app development.
2. Understanding the React Native Interview Process
The React Native interview process typically consists of several stages, each designed to assess different aspects of a candidate’s abilities and fit for the role. Initially, candidates may encounter a screening call or interview, which often includes questions about their experience and background in React Native and mobile development. This is a chance for the interviewer to gauge the applicant’s communication skills and enthusiasm for the position.
Following the screening, candidates should be prepared for a technical interview that delves into their knowledge of React Native. This could involve theoretical questions, practical coding problems, or both. It’s common for interviewers to ask about React Native concepts such as components, state management, and lifecycle methods. They may also test understanding of broader concepts like functional and class components, React hooks, and context API.
Some organizations include a take-home coding challenge that allows candidates to demonstrate their problem-solving skills in a less pressurized environment. This stage is crucial for showcasing your ability to write clean, efficient code and to solve real-world problems using React Native.
Moreover, candidates may face system design interviews, where they’re expected to architect a scalable and performant application using React Native. This assesses the applicant’s ability to make decisions that affect the codebase and product over the long term.
Finally, the interview process often concludes with an interview focused on behavioral questions and cultural fit. This is the opportunity for interviewers to understand how a candidate would mesh with the team and company values. It also allows the candidate to express their own goals and interests to ensure a mutual fit.
Throughout the interview process, communication skills are essential. Being able to articulate your thought process and solutions clearly can be just as important as the technical skills themselves. Preparing for each stage of the interview process with a comprehensive understanding of React Native and a reflection on past experiences will be key to success.
3. Fundamental React Native Concepts You Should Know
Understanding fundamental React Native concepts is crucial for any developer aiming to excel in a React Native interview. First and foremost, it’s essential to have a firm grasp of React Native’s core building blocks: components. Components are the heart of any React Native application, and understanding both functional and class components, along with their lifecycle methods, is key. Functional components, often used with React hooks like useState and useEffect, have become more prevalent with the introduction of React hooks.
State management is another vital concept. State in React Native determines how components render and behave; as such, knowing how to handle state using the useState hook or stateful class components is fundamental. Props (short for “properties”) are equally important as they allow for the passing of data and event handlers between components, enabling component reuse and composition.
Navigation is a concept that often comes up in interviews, as mobile apps typically consist of multiple screens. Being familiar with popular navigation libraries such as React Navigation and understanding how to implement navigation stacks, tabs, and drawers is useful.
Performance optimization also plays a significant role in React Native development, and interviewers may look for knowledge in this area. This includes understanding the importance of using the PureComponent or React.memo for preventing unnecessary renders, knowing how to optimize list rendering with FlatList or SectionList, and being aware of common performance pitfalls and how to avoid them.
Lastly, interviewers may expect candidates to be familiar with connecting to APIs using fetch or axios, handling asynchronous operations with async/await or Promises, and integrating third-party libraries and native modules.
Building a strong foundation in these fundamental React Native concepts will not only help in passing interviews but will also provide a solid base for developing efficient and maintainable mobile applications with React Native.
4. Mastering React Native Components and Lifecycle
Mastering React Native components and their lifecycle is a critical part of developing robust mobile applications. Components are the building blocks of React Native apps, and understanding how they work is essential for creating dynamic and responsive user interfaces.
There are two types of components in React Native: functional components and class components. Functional components are simpler and used for presenting static data, whereas class components are more complex and allow for managing state and lifecycle methods.
Lifecycle methods are hooks that allow you to run code at specific points in a component’s life. For class components, these methods include componentDidMount
, componentDidUpdate
, and componentWillUnmount
. They’re used for tasks like making network requests, setting up subscriptions, and optimizing performance.
The introduction of hooks in React Native has shifted the focus towards functional components for many developers. Hooks like useState
and useEffect
give functional components the ability to use state and side effects, which were previously only available in class components. Understanding how to use these hooks effectively is now an indispensable part of React Native development.
Components must also be designed to handle props correctly. Props are read-only and allow parent components to pass data down to their children. Knowing how to use props to create reusable and composable components is a fundamental skill.
Another aspect of mastering components is the concept of conditional rendering. React Native developers must know how to render different UI elements based on certain conditions, such as user actions or data changes.
In any React Native interview, you may be asked to explain how you would build a component or to discuss the best practices for managing a component’s lifecycle. Therefore, having a solid grasp of these concepts and the ability to articulate them clearly is crucial. Demonstrating a deep understanding of components and lifecycle methods will show potential employers that you have the technical expertise necessary to build high-quality React Native applications.
5. Handling State and Props in React Native
Handling state and props effectively is fundamental to React Native development, as they are key elements in creating interactive and dynamic applications. State represents the mutable data within a component, while props are the immutable data passed from parent to child components, enabling components to communicate with each other.
In managing state, React Native developers typically use the useState
hook within functional components, which allows for state variables to be maintained across re-renders. For class components, state is managed through the state object and is modified via the setState
method. It’s important to understand when and why to use local state and when to elevate state to higher-level components or even use state management libraries like Redux or Context API for more complex state management across the entire application.
Props, on the other hand, are used to pass data and event handlers down to child components. They are read-only within the child component that receives them, and any modifications to prop values should occur in the parent component that owns the state. One common interview topic might be the concept of “lifting state up” where state is moved to higher-level components to facilitate sharing across multiple children components.
Another important aspect is understanding the concept of “prop drilling” and how to avoid it. Prop drilling refers to the process of passing props through multiple levels of components, which can lead to maintenance issues and less readable code. To circumvent this, React offers advanced patterns and tools like context or state management libraries.
Moreover, developers should be familiar with the principles of immutability with regards to state and props, as mutating state directly can lead to bugs and unpredictable behavior in React Native applications. Instead, developers should use setState or the setter function from useState
to create new objects or arrays when updating state.
During an interview, you may be asked to explain how to handle state and props, how to pass props correctly, or to describe a situation where you had to lift state up. Being able to articulate your approach to state and props management, with examples from your past projects, will demonstrate your proficiency in building React Native applications that are both efficient and maintainable.
6. Important React Native Libraries and Tools
Familiarity with key React Native libraries and tools is essential for any developer looking to excel in this framework. These libraries and tools can significantly streamline the development process, enhance app functionality, and improve the overall performance and maintainability of the applications.
One of the most important libraries in the React Native ecosystem is React Navigation, which provides a straightforward and customizable solution for navigating between the screens of your application. It supports stack navigation, tab navigation, drawer navigation, and more, making it a versatile choice for implementing navigation in your app.
For state management, libraries such as Redux and MobX are commonly used. Redux offers a predictable state container that makes it easier to manage the state of your app, while MobX provides a simpler and more scalable approach to state management through reactive programming.
When it comes to UI components and design, libraries like NativeBase and React Native Elements provide pre-built components that can be easily customized to fit the design requirements of your app. These libraries help speed up the development of attractive and responsive interfaces.
For networking and handling API calls, Axios is a promise-based HTTP client that’s popular among React Native developers. It simplifies the process of sending asynchronous HTTP requests to REST endpoints and performing CRUD operations.
To enhance performance, tools like Hermes, a JavaScript engine optimized for running React Native, can be used to improve startup times and reduce memory usage on Android devices.
Another crucial tool is Flipper, a debugging platform for mobile apps that supports React Native. It provides valuable insights into your app’s performance and can help you diagnose and fix issues more efficiently.
Testing libraries such as Jest for unit testing and Detox for end-to-end testing are also important for maintaining high code quality and ensuring that your app works as expected.
Lastly, for continuous integration and delivery, tools like Fastlane and Bitrise are popular choices among React Native developers. These tools automate the process of building, testing, and deploying your mobile apps, which can greatly enhance the workflow and productivity of development teams.
Being well-versed in these libraries and tools not only prepares you for common React Native interview questions but also demonstrates your commitment to professional development and your capability to leverage the best practices in the field. It’s advisable to have hands-on experience with these tools and be able to discuss how you’ve used them in past projects during your interview.
7. Navigating React Native APIs and Performance Optimization
Navigating React Native APIs and optimizing performance are critical skills for developers to ensure that applications not only function correctly but also deliver a smooth user experience.
React Native provides a set of APIs for accessing device and platform functionality, such as camera, geolocation, and local storage. Understanding how to work with these APIs is crucial for building fully-featured apps. For example, you may need to use the AsyncStorage API to store data locally on the user’s device or the Geolocation API to obtain the user’s current position.
Performance optimization is another key area of focus. React Native applications can suffer from performance issues if not properly optimized. Developers should be familiar with strategies to optimize performance, such as reducing the size of the app bundle by using tools like Proguard, enabling Hermes on Android for improved execution speed, and optimizing images and other assets.
One common performance bottleneck in React Native applications is inefficient rendering. Proficient developers should know how to use the shouldComponentUpdate
lifecycle method or React.memo
for functional components to prevent unnecessary re-renders. They should also be aware of the performance implications of inline functions and the use of the useCallback
hook to memoize callbacks.
Another important aspect of performance optimization is the effective use of lists. The FlatList
and SectionList
components in React Native are optimized for long lists of data and help to recycle list items that scroll off the screen, reducing memory usage and improving scrolling performance.
During an interview, you might be asked to discuss your experience with React Native’s APIs and your approach to optimizing performance in a past project. You should be prepared to explain specific strategies you have used to overcome performance challenges, such as implementing lazy loading of images, using efficient data structures, and minimizing the use of heavy computations in the render method.
Having a solid understanding of how to navigate React Native APIs and optimize performance will not only make your applications stand out but also demonstrate your technical expertise and problem-solving abilities to potential employers.
8. Common React Native Interview Questions and Answers
React Native interviews often include a mix of technical questions that test your understanding of the framework and practical scenarios to assess your problem-solving abilities. Below are some common interview questions along with insightful answers that could help you prepare:
Question: What is the difference between state and props in React Native?
Answer: State is a mutable object that determines the behavior and rendering of components and is managed within the component, while props are immutable and passed from parent to child component to configure or share data.Question: How do you improve the performance of a React Native application?
Answer: To improve performance, you can optimize render methods using PureComponent or React.memo, use theshouldComponentUpdate
method oruseCallback
anduseMemo
hooks to avoid unnecessary renders, effectively manage state, and use theFlatList
orSectionList
for efficient list rendering. Enabling the Hermes engine on Android can also boost performance.Question: Explain the purpose of the Flexbox layout in React Native.
Answer: Flexbox is a layout system that allows for a flexible and responsive layout in React Native apps. It enables developers to define how components should be positioned and sized within a container, adapting to different screen sizes and orientations.Question: How do you handle asynchronous operations in React Native?
Answer: Asynchronous operations in React Native can be handled using JavaScript’s async/await syntax, Promises, or libraries like Redux-Saga or Redux-Thunk for more complex asynchronous flows involving state management.Question: What is the significance of keys in lists?
Answer: Keys help React Native identify which items have changed, are added, or are removed, which is important for performance and efficient update of lists. Each item in a list should have a unique key to prevent rendering issues and optimize re-rendering.Question: How do you navigate between screens in a React Native app?
Answer: Navigation between screens is managed using navigation libraries like React Navigation, which provides a variety of navigators such as stack, tab, and drawer navigators to handle the transition between different screens in an app.Question: Can you explain what React Native linking is?
Answer: Linking is a process that allows apps to interact with other apps or websites. It can be used to open a web URL or to deep link into another app by specifying a custom URL scheme.Question: Describe the use of higher-order components in React Native.
Answer: Higher-order components (HOCs) are functions that take a component and return a new component with additional props or behavior. They are used for reusing component logic, such as connecting to a Redux store or providing theme context.
By understanding these questions and formulating clear, concise answers, you can demonstrate your knowledge of React Native and your ability to address common challenges faced in mobile app development. It’s important to not only memorize answers but also understand the underlying concepts so you can adapt to variations of these questions or solve related problems during your interview.
9. Tips for Effective Communication During Your Interview
Effective communication during your React Native interview is as crucial as your technical skills. Here are some tips to help you communicate more effectively:
Articulate Your Thought Process: When solving problems or answering questions, explain your thought process clearly. This shows interviewers how you approach problems and that you can logically navigate through challenges.
Listen Actively: Pay close attention to the questions being asked. If something is unclear, don’t hesitate to ask for clarification. This demonstrates your attention to detail and ensures you’re answering the question correctly.
Use Technical Terminology Appropriately: While it’s important to demonstrate your technical knowledge, make sure to explain concepts in a way that is understandable, avoiding excessive jargon that may confuse non-technical interviewers.
Show Enthusiasm: Express your passion for React Native and mobile development. Your excitement about the role and the technology can be contagious and leave a positive impression.
Be Concise and Direct: Provide answers that are to the point. Avoid rambling, which can make it seem like you’re unsure of your answer. If you don’t know the answer to a question, it’s better to be honest rather than trying to fill the silence with irrelevant information.
Discuss Past Experiences: When discussing your background, focus on relevant experiences that showcase your skills and achievements with React Native. Use specific examples to illustrate how you’ve applied your knowledge in real-world situations.
Demonstrate Problem-Solving Skills: When presented with a problem during the interview, walk the interviewer through your problem-solving approach. Discuss the steps you would take to find a solution, highlighting your analytical and debugging skills.
Be Positive About Past Experiences: Speak positively about past projects and employers. Focus on what you learned and how you’ve grown as a developer, rather than on negative experiences.
Show Curiosity: Ask insightful questions about the company’s projects, technologies, and culture. This not only shows you’ve done your homework but also that you’re considering how you can contribute to and grow with the company.
By following these tips, you can improve the quality of your communication during your React Native interview. Good communication can set you apart from other candidates by demonstrating that you not only have the technical know-how but also the soft skills necessary to be an effective team member.
10. Building a Portfolio and Showcasing Your React Native Projects
Building a portfolio and showcasing your React Native projects is an essential step in demonstrating your capabilities to potential employers. A well-constructed portfolio provides a tangible way for interviewers to assess your technical skills, problem-solving abilities, and creativity.
When building your portfolio, include a variety of projects that display your proficiency with React Native. These can range from simple applications that demonstrate fundamental concepts to more complex projects that highlight your ability to build scalable, production-ready applications. Ensure each project is accompanied by a thorough explanation of the technologies used, the challenges faced, and the solutions implemented.
Your portfolio should be easily accessible, preferably hosted online on platforms like GitHub or GitLab, where your code can be reviewed. Make sure the code is clean, well-documented, and follows best practices. Providing live demos or links to the published apps in the App Store or Google Play can make a stronger impact than just code repositories.
In addition to the projects themselves, include case studies or project write-ups that delve deeper into your development process. Discuss the objectives of the project, your design decisions, and how you tackled specific technical hurdles. This narrative can give employers insight into your approach to app development and your ability to communicate complex ideas effectively.
Furthermore, it’s beneficial to contribute to open-source projects or community libraries, as this demonstrates your engagement with the wider developer community and your continuous learning and improvement. Highlighting any contributions, you’ve made can set you apart from other candidates.
It’s also important to tailor your portfolio to the job you’re applying for. Emphasize the projects and skills that are most relevant to the role. If the position requires a strong understanding of UI/UX design, for instance, showcase projects where you implemented exceptional user interfaces.
Lastly, ensure your portfolio reflects your current skill level. Regularly update it with new projects or enhancements to existing ones. This not only shows your dedication to your craft but also that you’re keeping up with the latest trends and advancements in React Native development.
By carefully selecting and presenting your React Native projects, you can create a compelling portfolio that not only showcases your technical expertise but also tells the story of your development journey and your passion for mobile app development.
11. The Role of Coding Challenges in React Native Interviews
Coding challenges play a significant role in React Native interviews as they provide interviewers with a practical way to evaluate a candidate’s technical skills, problem-solving abilities, and coding style. These challenges often take the form of algorithmic questions, debugging exercises, or building a small feature within a limited time frame.
During a React Native interview, a coding challenge may require you to demonstrate proficiency with the framework’s components, state management, and other fundamental concepts. It’s not just about solving the problem, but also about how you approach the task, how you structure your code, and your ability to write clean, efficient, and maintainable code.
These challenges help interviewers assess your familiarity with React Native’s syntax and best practices. For example, you might be asked to create a component with certain functionality, manage the component’s state effectively, or integrate with APIs. Your performance in these exercises can give interviewers insight into how you would handle real-world programming scenarios.
Additionally, coding challenges can reveal your thought process when faced with bugs or when you need to implement a feature that you may not be immediately familiar with. How you deal with ambiguity, your research skills, and your resilience in the face of challenging problems are all important qualities that can be observed during these exercises.
It’s beneficial to prepare for coding challenges by practicing with online coding platforms and familiarizing yourself with common algorithms and data structures. Being comfortable with the mechanics of coding under time constraints will help you remain calm and focused during the interview.
Moreover, your ability to clearly explain your code and the reasoning behind your decisions is crucial. Interviewers will often be interested in a walkthrough of your solution, so practicing verbalizing your thought process can be as important as the coding itself.
While coding challenges are a common part of the interview process, they are typically just one component. They are combined with other types of interview questions to provide a comprehensive view of your abilities. Therefore, a balanced preparation that includes both technical practice and soft skills development is key to succeeding in React Native interviews.
12. Preparing for Behavioral Questions and Company Culture Fit
Preparing for behavioral questions and assessing company culture fit are crucial components of the interview process for any React Native developer role. Behavioral questions are designed to understand how you work, think, and interact with others in various professional scenarios. These questions often start with phrases like “Tell me about a time when…” or “Give me an example of how you…”.
To prepare for these questions, reflect on your past work experiences and identify instances where you demonstrated key skills such as teamwork, leadership, problem-solving, communication, and adaptability. Use the STAR method (Situation, Task, Action, Result) to structure your responses, ensuring you convey the context, your role, the actions you took, and the outcomes.
Interviewers use behavioral questions to gauge how you might fit within the company’s culture. Every company has its own set of values and ways of working, so it’s important to do your research beforehand. Look into the company’s mission statement, core values, and recent news to understand their culture and business goals. This will help you tailor your answers to align with their philosophy and show that you’re not only a good technical fit but also a good cultural fit.
Additionally, being prepared with questions of your own about the company’s culture, team dynamics, and expectations can demonstrate your genuine interest in the role and the organization. It allows you to decide if the company is the right environment for you to thrive professionally.
Remember that behavioral questions are not just about what you’ve done in the past; they are also about how you convey those experiences. Practice delivering your answers with confidence, clarity, and sincerity. Your goal is to illustrate that you possess the soft skills necessary to be an effective team member and that your values align with those of the company.
Ultimately, showing that you are not only technically capable but also a good match for the company’s culture will help set you apart from other candidates and increase your chances of receiving a job offer.
13. Strategies for Negotiating Your Job Offer Post-Interview
Negotiating your job offer post-interview is a critical step in your job search process, and it requires a strategic approach to ensure you get the best possible package. Here are some effective strategies:
Understand Your Worth: Before entering negotiations, research industry standards for React Native developer positions in your location and with your level of experience. Understand the value you bring to the company and be prepared to articulate it.
Evaluate the Entire Offer: Look beyond the salary. Consider other aspects of the offer such as health benefits, retirement plans, vacation time, remote work options, professional development opportunities, and any other perks. Determine what is most important to you.
Express Enthusiasm: Start the negotiation by expressing your excitement about the opportunity and your eagerness to contribute to the company. This sets a positive tone for the discussion.
Be Prepared to Articulate Your Case: Use specific examples from your past work experience to demonstrate why you deserve a higher offer. Highlight your skills, accomplishments, and how they align with the company’s needs.
Practice Negotiation Techniques: Role-play negotiations with a friend or mentor to build your confidence. Practicing can help you refine your approach and prepare for potential counterarguments.
Consider Timing: Don’t rush into negotiations immediately after receiving the offer. Take the time to thoroughly review it and prepare your response. However, avoid delaying too long, as it may signal a lack of interest.
Communicate Clearly and Professionally: Whether negotiating in person, over the phone, or via email, keep the tone professional. Be clear about what you are asking for and why you believe it’s justified.
Be Flexible: Negotiations are a two-way street. Be open to creative solutions that might involve a trade-off between salary and other benefits. For example, if the company can’t meet your salary expectations, they might offer additional vacation days or a signing bonus.
Know When to Walk Away: It’s important to know your bottom line. If the company is unable to meet your minimum requirements and you have other options, it may be best to politely decline the offer.
Get Everything in Writing: Once you have come to an agreement, make sure the revised terms are documented in the official offer letter. This ensures there are no misunderstandings about what was agreed upon.
Negotiating can be challenging, but by being prepared and approaching the conversation with a clear, firm, and professional demeanor, you can significantly improve the terms of your job offer. Remember, the goal is to reach an agreement that’s satisfying for both you and the employer.
14. Final Checklist Before the React Native Interview
As you prepare for your React Native interview, going through a final checklist can ensure you’re fully prepared and confident. Here are the key items to review before the interview:
Review Core Concepts: Make sure you have a solid understanding of React Native’s fundamental concepts such as components, state, props, lifecycle methods, hooks, and navigation.
Brush Up on Latest Features: Stay current with the latest updates in React Native. Review any new features or changes that have been introduced since you last worked with the framework.
Practice Coding Problems: Go through a set of common React Native coding problems to refresh your problem-solving skills. Focus on areas where you feel less confident.
Revisit Your Projects: Be prepared to discuss your previous React Native projects in detail. Understand the challenges you faced, the solutions you implemented, and the impact of your work.
Prepare for Behavioral Questions: Reflect on your past experiences and come up with examples that showcase your problem-solving skills, teamwork, and adaptability. Practice articulating these experiences using the STAR method.
Understand the Company: Research the company you are interviewing with. Understand their products, culture, and the role you’re applying for. Think of questions to ask that demonstrate your interest in the company and the position.
Review the Job Description: Look over the job description again and make a note of any specific skills or experiences that are emphasized, so you can be sure to highlight these during the interview.
Mock Interviews: If possible, conduct a mock interview with a friend or mentor to practice your responses and get feedback.
Prepare Your Environment: If the interview is virtual, test your technology beforehand. Ensure your webcam, microphone, and internet connection are working properly. Choose a quiet, well-lit space for the interview.
Dress Appropriately: Decide what to wear in advance. Even for virtual interviews, dressing professionally can help put you in the right mindset.
Pack Your Bag: If you’re interviewing in person, pack everything you need the night before. Include extra copies of your resume, a portfolio of your work, a list of references, and any other materials you may need to present.
Plan Your Route: If the interview is on-site, plan your route and consider any potential delays. Aim to arrive a little early to account for unexpected issues.
Relax and Get Rest: Try to relax the evening before the interview. Avoid last-minute cramming and get a good night’s sleep to ensure you are well-rested and alert.
By following this checklist, you’ll be well on your way to making a great impression during your React Native interview. Good luck!
15. Conclusion: Staying Updated with React Native Trends
Staying updated with React Native trends is a continuous process that is essential for any developer looking to remain competitive in the field of mobile application development. As React Native evolves, new updates, libraries, and best practices emerge, making it important to stay abreast of the latest advancements.
Engaging with the React Native community is one of the most effective ways to stay informed. Participating in forums, attending meetups, and joining online communities, such as React Native’s GitHub repository or Discord channels, can provide insights into emerging trends and common challenges faced by developers.
Following thought leaders and contributors on social media platforms like Twitter or subscribing to newsletters and blogs focused on React Native can also keep you updated. Additionally, attending conferences and webinars is a great way to learn from experts and network with peers.
Another key aspect is to contribute to open-source projects or create your own. This not only helps you apply new concepts and stay active in the community but also gets you direct feedback from other developers, which can be invaluable for your professional growth.
Regularly revisiting the official React Native documentation, as well as keeping an eye on the React Native roadmap, will alert you to upcoming changes and deprecations that could affect your current or future projects.
Lastly, dedicating time to continuous learning through online courses, tutorials, and hands-on experimentation with new features and tools will ensure that your skills remain current and marketable.
By committing to lifelong learning and staying engaged with the React Native community, developers cannot only keep pace with the industry but also anticipate changes that can lead to more innovative and efficient app development.