Software Services
For Companies
Portfolio
Build With Us
Table of Contents:
How to Build a CMS with Laravel/

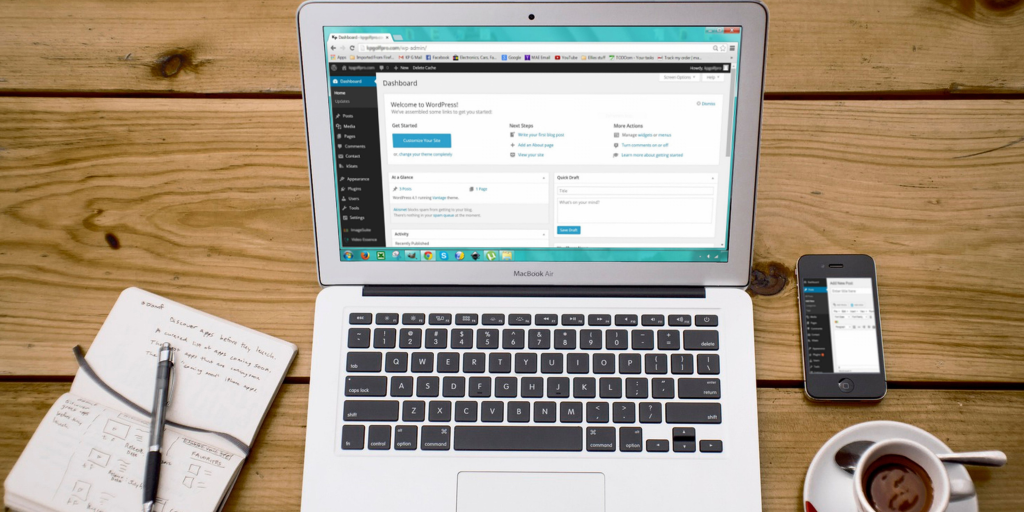
A content management system (CMS) is a powerful tool for managing and publishing web content. With a CMS, users can create, edit, and organize content without any knowledge of HTML or other programming languages. In this article, we’ll explore how to build a CMS using Laravel, a PHP web application framework.
Why Choose Laravel?
Laravel is a popular PHP framework known for its simplicity, elegance, and robust features. It offers a wide range of tools and libraries that make web development faster and more efficient. Laravel’s modular design, combined with its easy-to-learn syntax and well-organized documentation, makes it an ideal choice for building a CMS.
Building a CMS with Laravel
Before diving into the details of building a CMS with Laravel, it’s important to understand the basic structure and functionality of a CMS. A CMS consists of two main components: a back-end or administrative panel, where content is managed, and a front-end, where content is displayed to users. With that in mind, here’s how you can build a CMS with Laravel:
Step 1: Install Laravel
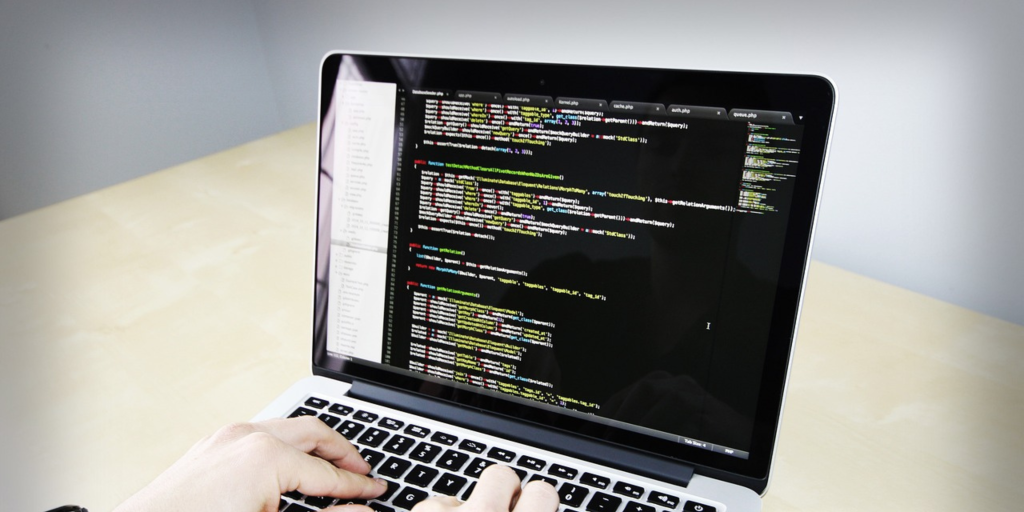
The first step in building a CMS with Laravel is to install Laravel itself. Laravel provides a convenient installer that can be accessed via Composer, a PHP package manager. Once you have Composer installed, open up your terminal and type the following command:
“`
composer create-project –prefer-dist laravel/laravel cms
“`
This will install Laravel in a directory called “cms”. You can replace “cms” with whatever name you’d like for your project.
Step 2: Set Up the Database
After installing Laravel, you’ll need to set up the database for your CMS. Laravel supports a wide range of databases, including MySQL, PostgreSQL, and SQLite. To configure the database, open the .env file located in the root directory of your project and enter your database credentials. For example:
“`
DB_CONNECTION=mysql
DB_HOST=127.0.0.1
DB_PORT=3306
DB_DATABASE=your_database_name
DB_USERNAME=your_database_username
DB_PASSWORD=your_database_password
“`
Step 3: Create the Models
Once the database is set up, you’ll need to create the models that will represent the different types of content in your CMS. In Laravel, models are PHP classes that interact with the database. To create a model, use the Artisan command:
“`
php artisan make:model Post
“`
This will create a new model called “Post”. You can create additional models for other types of content, such as pages or images, using the same command.
Step 4: Define the Database Schema
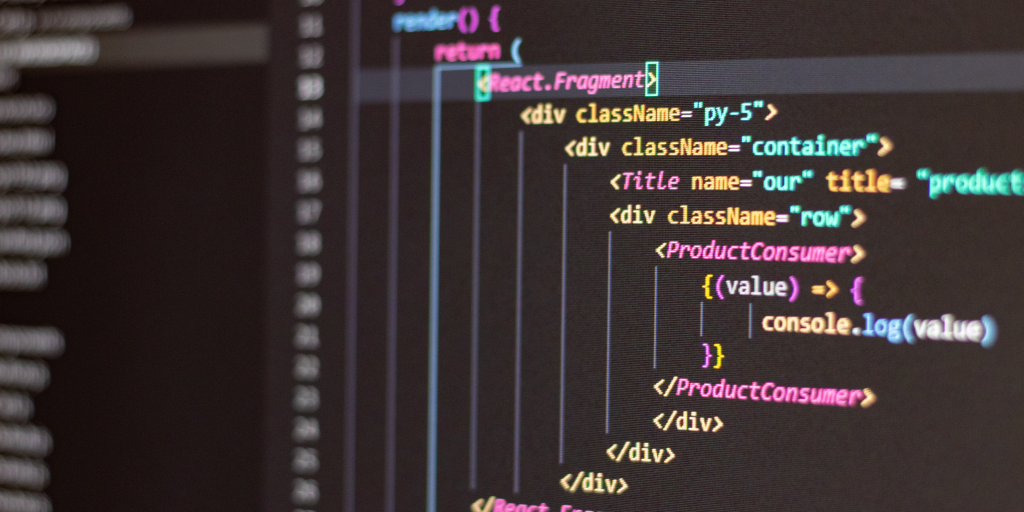
After creating the models, you’ll need to define the database schema for each model. Laravel provides a convenient way to define database schema using migrations. Migrations are PHP files that describe the changes you want to make to the database. To create a migration for the “posts” table, use the Artisan command:
“`
php artisan make:migration create_posts_table –create=posts
“`
This will create a new migration file in the “database/migrations” directory. Open up the migration file and define the schema for the “posts” table. For example:
“`
public function up()
{
Schema::create(‘posts’, function (Blueprint $table) {
$table->id();
$table->string(‘title’);
$table->text(‘body’);
$table->timestamps();
});
}
public function down()
{
Schema::dropIfExists(‘posts’);
}
“`
Once you have set up the necessary routes and controllers, you can start building the views that will allow you to manage your content. Laravel’s Blade templating engine is a great tool for this, allowing you to create reusable templates and components.
To create a new page in your CMS, you can create a new Blade template file in the `resources/views/pages` directory. You can then define the HTML structure of your page and include placeholders for dynamic content using Laravel’s Blade syntax.
For example, if you want to display a list of blog posts on a page, you can define a loop in your template that will iterate over an array of posts retrieved from the database. You can then use Blade’s `@foreach` directive to generate HTML for each post.
“`
@foreach ($posts as $post)
<div class=”post”>
<h2>{{ $post->title }}</h2>
<p>{{ $post->body }}</p>
</div>
@endforeach
“`
You can also use Blade’s conditional statements and partials to create more complex templates. For example, you can include a separate partial for the header and footer of your website and use the `@include` directive to include them in your page templates.
Once you have created your templates, you can link them to your controllers using routes. Laravel’s `Route::get` method allows you to define a route that will call a specific controller method when it is accessed.
For example, you can define a route for the blog page that will call the `BlogController@index` method when it is accessed:
“`
Route::get(‘/blog’, ‘BlogController@index’);
“`
In your `BlogController` class, you can define the `index` method to retrieve the necessary data from the database and pass it to the view:
“`
public function index()
{
$posts = Post::all();
return view(‘pages.blog’, [‘posts’ => $posts]);
}
“`
This method retrieves all blog posts from the `posts` table and passes them to the `pages.blog` view. The `pages.blog` view can then use the `@foreach` directive to display the posts.
Conclusion
In this article, we have explored how to build a CMS with Laravel. We started by setting up a new Laravel project and installing the necessary packages. We then created a database and set up the necessary models and migrations to manage our content.
We also discussed how to create routes and controllers to manage the different sections of our CMS, as well as how to use Laravel’s Blade templating engine to create dynamic views.
By following these steps, you can create a powerful CMS that allows you to manage your content and publish it to your website with ease. Laravel’s powerful tools and elegant syntax make it a great choice for building web applications of all types, from simple blogs to complex e-commerce platforms.