Software Services
For Companies
For Developers
Portfolio
Build With Us
Table of Contents:
Mastering Multithreading in Node.js: A Comprehensive Guide/

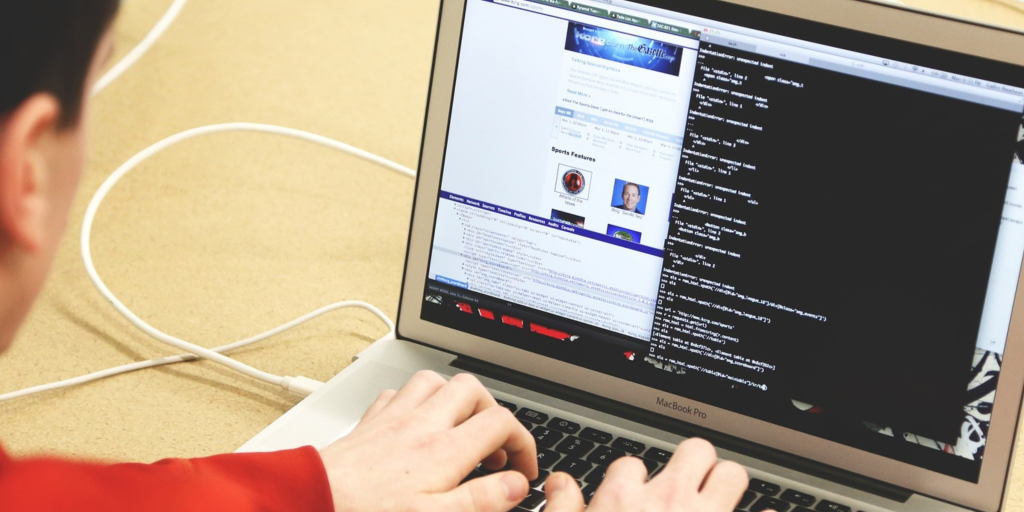
Node.js has revolutionized the world of server-side JavaScript, providing developers with an efficient and scalable platform. While Node.js is inherently single-threaded, it’s possible to leverage multithreading techniques to enhance performance and handle concurrent tasks effectively. In this guide, we’ll explore how to harness the power of multithreading in Node.js to build high-performance applications.
Understanding Node.js’s Single-Threaded Nature
Node.js is built on the V8 JavaScript engine, which employs a single-threaded event loop to handle asynchronous tasks. While this design is efficient for I/O-bound operations, it can become a bottleneck when dealing with compute-intensive tasks. Multithreading can address this limitation and enable Node.js to make better use of available CPU cores.
The Worker Threads Module: Introducing Multithreading
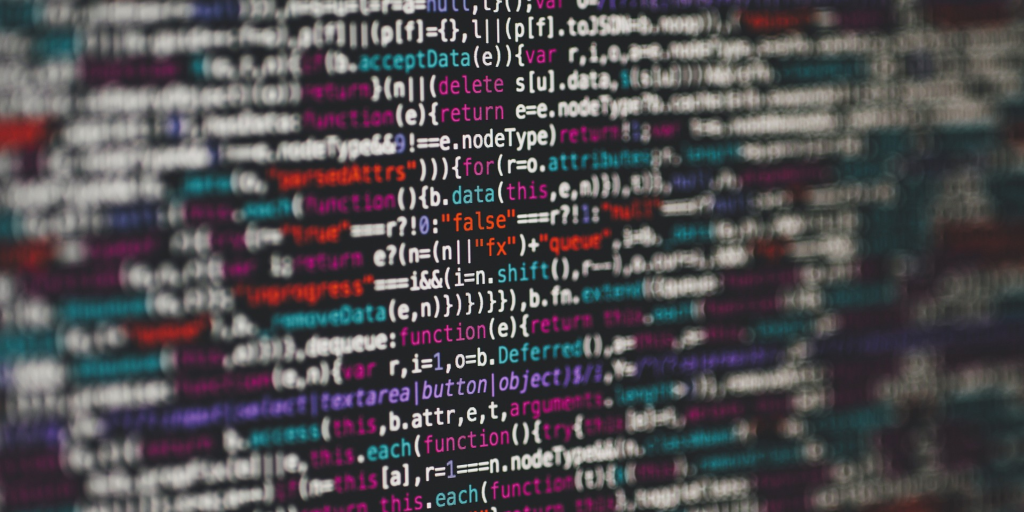
Node.js introduced the worker_threads
module to enable multithreading. This module allows developers to create multiple worker threads that can execute tasks in parallel, utilizing multiple CPU cores. This approach is particularly useful for computationally intensive tasks, such as image processing or data analysis.
Benefits of Multithreading in Node.js
- Improved Performance: By distributing tasks across multiple threads, Node.js can achieve better CPU utilization and reduce processing times, leading to improved application performance.
- Responsive Applications: Multithreading prevents a single compute-intensive task from blocking the event loop, ensuring that the application remains responsive to user interactions.
- Parallel Processing: Multithreading enables simultaneous execution of tasks, making it possible to handle multiple tasks concurrently and efficiently.
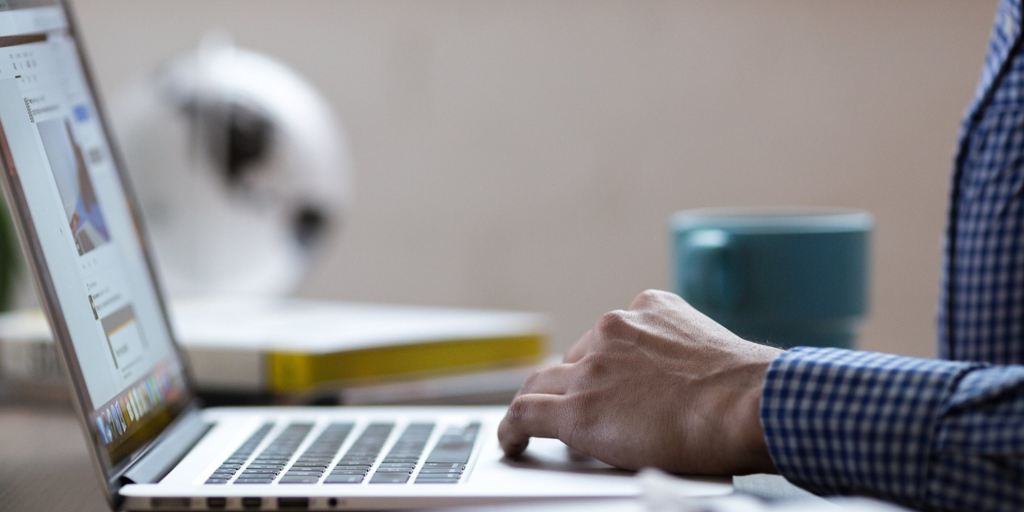
Implementing Multithreading in Node.js
- Creating Worker Threads: To implement multithreading, import the
worker_threads
module and create worker threads using theWorker
class. Each worker thread operates independently and can communicate with the main thread through message passing. - Message Passing: Worker threads communicate with the main thread by sending and receiving messages. The
postMessage()
method is used to send messages from the main thread to a worker, while theon('message')
event listener is used to receive messages in the worker thread. - Shared Data: While worker threads operate in isolation, it’s possible to share data between threads using
SharedArrayBuffer
andAtomics
objects. This allows for coordinated access to shared memory, ensuring data consistency.
Use Cases for Multithreading in Node.js
- Image Processing: Multithreading is ideal for tasks like image resizing or applying filters to images, where each image can be processed in a separate worker thread.
- Data Analysis: When dealing with large datasets, multithreading can accelerate data analysis tasks by distributing data chunks to different worker threads.
- Cryptographic Operations: Multithreading can speed up cryptographic tasks that involve heavy computations, such as hashing or encryption.
Best Practices for Multithreading in Node.js
- Task Granularity: Divide tasks into appropriately sized chunks to maximize parallel processing and avoid thread contention.
- Resource Management: Monitor the number of active threads to prevent resource exhaustion. Overloading with threads can lead to reduced performance.
- Error Handling: Implement error handling mechanisms to ensure that errors in worker threads are caught and appropriately managed.
Challenges and Considerations
- Synchronization: When multiple threads access shared resources, synchronization mechanisms like locks and semaphores should be used to prevent data corruption.
- Debugging: Debugging multithreaded applications can be complex. Use tools like the
inspector
module and debuggers to identify and fix issues.
Conclusion
Multithreading in Node.js opens up new possibilities for building high-performance applications that can handle compute-intensive tasks efficiently. By understanding the worker_threads
module, implementing message passing, and leveraging shared data, developers can harness the full potential of multithreading in Node.js. As Node.js continues to evolve, mastering multithreading will become an essential skill for building responsive and scalable applications.
Stay up-to-date with the latest trends in Node.js and multithreading by visiting slashdev.io
For more insights and practical tutorials on Node.js and multithreading, visit slashdev.io to explore a wealth of resources that can help you optimize your development workflows and build cutting-edge applications.