Software Services
For Companies
For Developers
Portfolio
Build With Us
Get Senior Engineers Straight To Your Inbox

Every month we send out our top new engineers in our network who are looking for work, be the first to get informed when top engineers become available

At Slashdev, we connect top-tier software engineers with innovative companies. Our network includes the most talented developers worldwide, carefully vetted to ensure exceptional quality and reliability.
Build With Us
NodeJS Interview Questions/
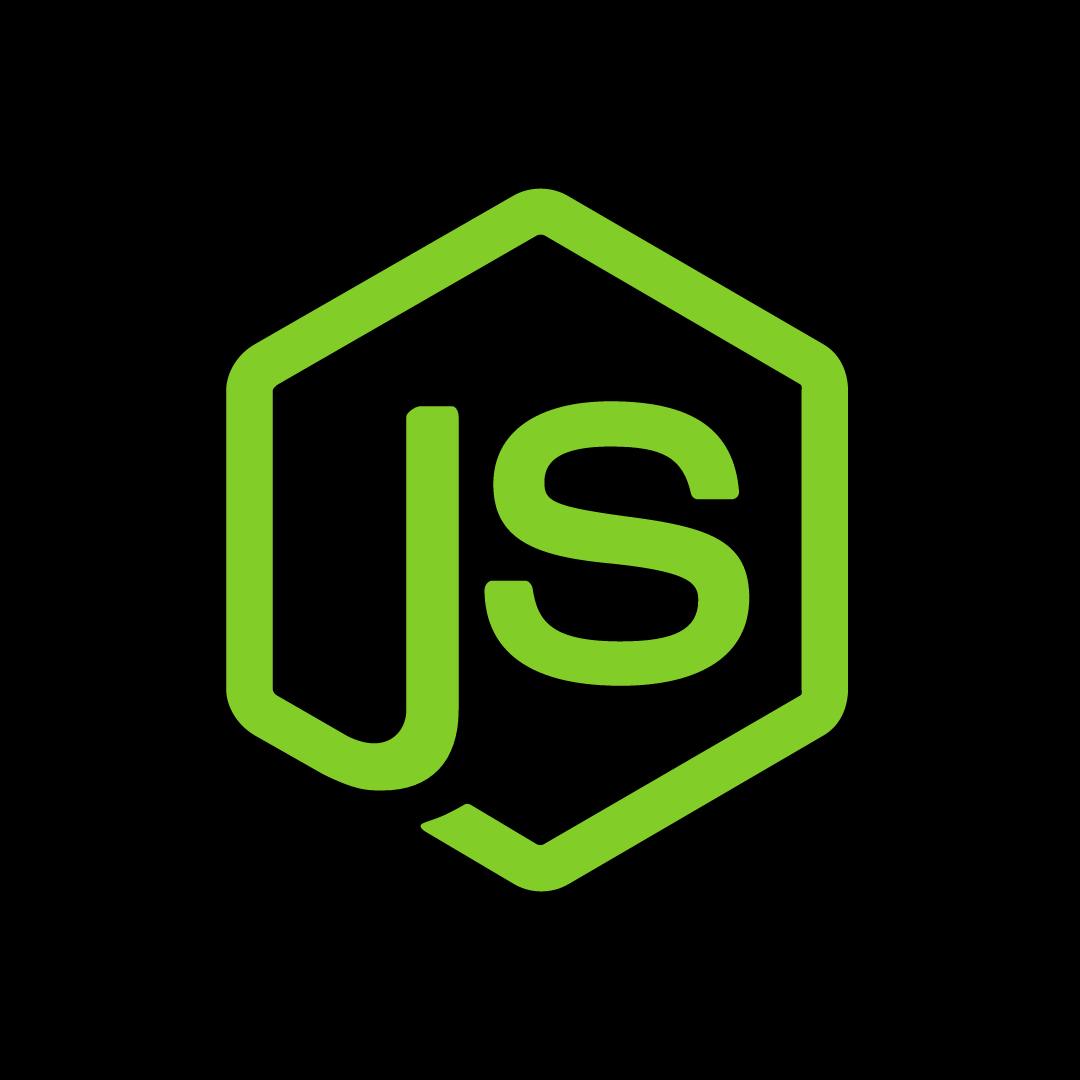
Below are some simple interview questions to ask an engineer to get an idea of their level of understanding of NodeJS and backend development. These are common questions we use in our interviews with engineers.
What is NodeJS and how does it work?/
Here we are looking to get an understanding of how well the engineer can explain the tool that they work with everyday. Something like the following could be good:
Node.js is a virtual machine that uses JavaScript as its scripting language and runs Chrome’s V8 JavaScript engine. Basically, Node.js is based on an event-driven architecture where I/O runs asynchronously making it lightweight and efficient. It is being used in developing desktop applications as well with a popular framework called electron as it provides API to access OS-level features such as file system, network, etc.
How do you use async await in NodeJS?/
// this code is to retry with exponential backoff
function wait (timeout) {
return new Promise((resolve) => {
setTimeout(() => {
resolve()
}, timeout);
});
}
async function requestWithRetry (url) {
const MAX_RETRIES = 10;
for (let i = 0; i <= MAX_RETRIES; i++) {
try {
return await request(url);
} catch (err) {
const timeout = Math.pow(2, i);
console.log('Waiting', timeout, 'ms');
await wait(timeout);
console.log('Retrying', err.message, i);
}
}
}
How do you write clean and maintainable NodeJS applications?/
In this question, we are trying to get an idea of the thought process of the engineer. Do they follow design principles like SOLID? Are they following a pattern for organizing their project? We want to see engineers who have a good methodology behind how they build project and the ability to talk to why they build it the way they do.