Software Services
For Companies
For Developers
Portfolio
Build With Us
Table of Contents:
Overview Of Building React Components/
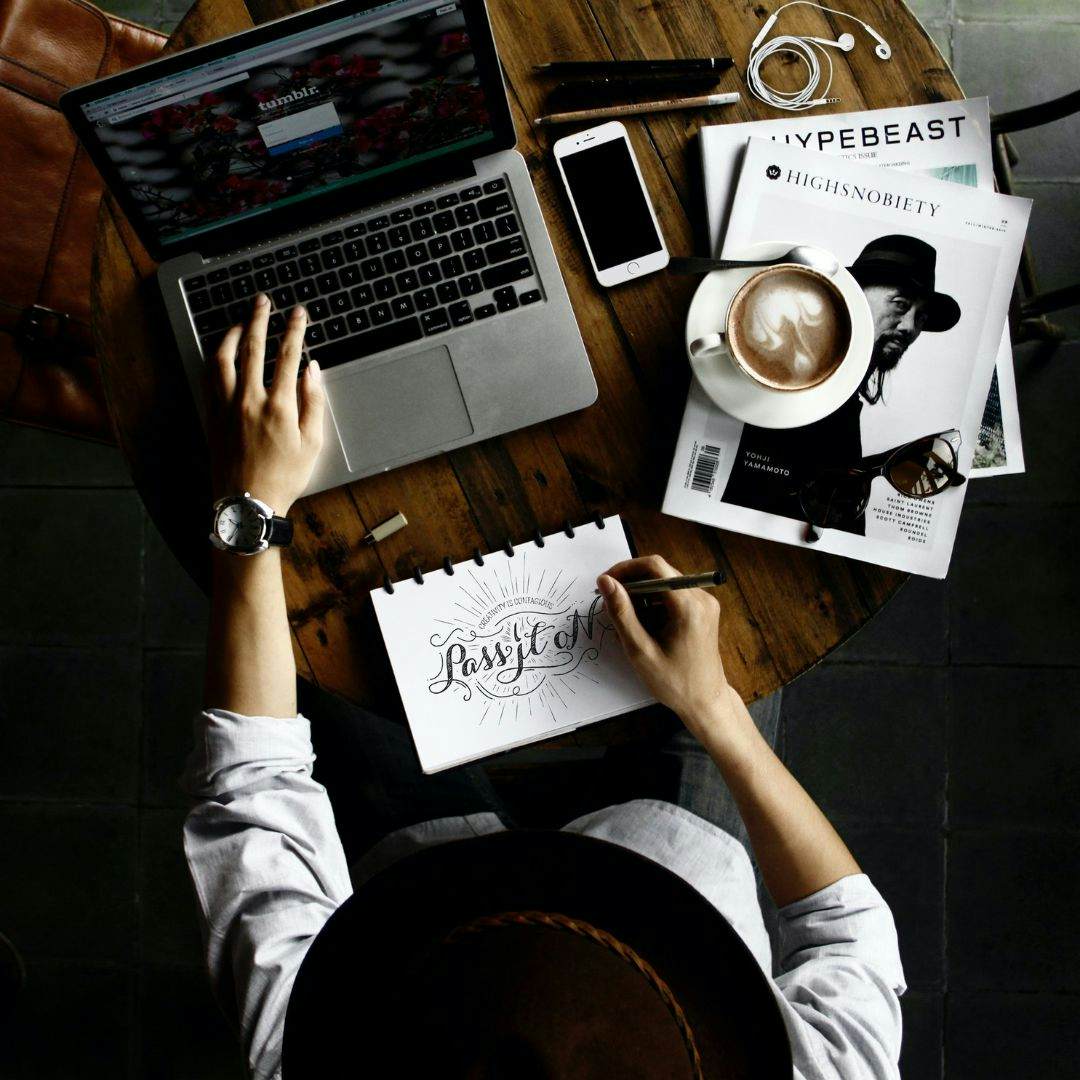
1. Introduction to React and Components
React is a popular JavaScript library for building user interfaces, particularly single-page applications where a fast and interactive user experience is essential. Developed by Facebook and maintained by a community of developers, React emphasizes declarative programming and the creation of reusable components, making it an efficient choice for developers looking to construct scalable and maintainable web applications.
At the heart of React’s philosophy is the concept of components. A component in React is a self-contained module that encapsulates all the logic, styling, and markup required to render a part of a user interface. Components can be as small as a button or as large as an entire application section, allowing developers to break down complex UIs into manageable, reusable pieces.
Components are typically defined using a mix of JavaScript and JSX (JavaScript XML), a syntax extension that allows you to write HTML-like code directly within your JavaScript files. This blend makes it easier to visualize the UI and connect it with the underlying data and behavior. Each component has its state and props – the state is internal data specific to the component, while props are external data passed down from parent components, enabling dynamic content rendering and interactive features.
React components come in two main varieties: class components and functional components. Class components, the traditional way of writing components, use ES6 classes and provide more features like lifecycle methods for managing a component’s creation, updating, and teardown. On the other hand, functional components are simpler, making use of hooks, a feature introduced in React 16.8, to handle state and side effects with less code and more straightforward composition.
The component-based architecture of React not only promotes a more organized and modular development approach but also aligns perfectly with modern web development practices. It encourages the DRY (Don’t Repeat Yourself) principle and can significantly improve project maintainability and ease of testing.
For those new to React or looking to enhance their web development skills, mastering the art of creating and using components is a vital step. Understanding how components interact and manage data is crucial for building reactive, efficient, and user-friendly applications that stand out in today’s fast-paced digital landscape.
2. Setting Up Your Development Environment
To set up a development environment conducive to SEO, there are several key steps you should take. This process ensures that your website or application is built from the ground up with search engine optimization in mind.
Firstly, choose a code editor that suits your needs and is popular among SEO professionals, such as Visual Studio Code or Atom. These editors offer SEO-friendly plugins that can help you optimize your code in real-time.
Next, you’ll want to install version control software, such as Git. This allows you to track changes, revert to earlier versions of your code, and collaborate with others without risking the integrity of your project.
It’s also crucial to have a local server environment on your machine. Tools like XAMPP, MAMP, or WAMP provide a local server stack, making it easier to test and debug your website or application without having to deploy it to a live server.
For SEO purposes, integrating an SEO analysis tool like Google Lighthouse or Screaming Frog SEO Spider into your development process is beneficial. These tools can scan your website to identify SEO issues, such as missing meta tags, ineffective keywords, or slow loading times.
Moreover, ensure that your development environment includes access to mobile testing tools. With the increasing importance of mobile-first indexing, it’s essential to check how your site performs on various devices and screen sizes.
Finally, familiarize yourself with a content management system (CMS) that is known for its SEO capabilities, such as WordPress, Drupal, or Joomla. These platforms often come with SEO plugins or modules that can expedite the optimization process.
By carefully setting up your development environment with these tools and practices, you can create a solid foundation for SEO success, ensuring that your projects are optimized from the start.
3. Understanding JSX: The Syntax of React
JSX, which stands for JavaScript XML, is a syntax extension for JavaScript that is commonly used with React to describe what the UI should look like. It allows you to write HTML structures in the same file as your JavaScript code, providing a way to structure component rendering without the need for concatenating strings or manually creating DOM elements.
One of the key aspects of JSX is its declarative nature, which makes the code easier to understand and debug. Instead of using traditional DOM manipulation to update the UI, you simply describe the UI state based on the underlying data model in your React components. When the data changes, React handles updating the UI to match this description.
JSX is not a requirement for using React, but it’s widely adopted because it visually resembles the layout of the UI that it generates, which can be more intuitive for developers, especially those with an HTML and CSS background. It also allows React to show more helpful error and warning messages, as it understands the layout of your UI.
Under the hood, JSX is transformed into JavaScript. When a React component is written using JSX, the JSX is transpiled into React.createElement
calls by tools like Babel. These calls create objects that represent the React elements, which React uses to construct the DOM and keep it up to date.
The syntax for JSX is similar to HTML with a few key differences. For instance, since JSX is closer to JavaScript, you’ll use camelCase property naming for attributes — so class
becomes className
and tabindex
becomes tabIndex
. Also, since JSX is embedded within JavaScript, you can use curly braces to interpolate JavaScript expressions directly within the JSX. This means you can embed variables, function calls, and even other React components within your JSX.
Another important aspect is that JSX tags can either represent HTML elements or React components. When a tag starts with a lowercase letter, it represents an HTML element, and when it starts with an uppercase letter, it signifies a React component.
JSX also enforces the rule that every element must be closed. For instance, even self-closing tags like <img>
and <input>
must be closed in JSX, typically with a trailing slash (<img />
, <input />
).
Understanding JSX is pivotal for any React developer as it provides a succinct and expressive way to create UI components. While it may seem unfamiliar at first, most developers quickly find that JSX syntax is easy to read and write, making the development process more efficient and enjoyable.
4. Functional Components vs. Class Components
When diving into the world of React, understanding the differences between functional components and class components is essential. Both serve as means to construct reusable pieces of your UI, yet they come with distinct characteristics and uses.
Functional components, also known as stateless components, are JavaScript functions that return JSX. They are the bread and butter of modern React development, especially after the introduction of hooks in React 16.8, which enable functional components to handle state and side effects. These components are favored for their simplicity and the absence of ‘this’ keyword, which can simplify the code and potentially reduce bugs. They are usually less verbose than class components and are easier to read and test due to their plain structure and functional nature.
On the other hand, class components, also referred to as stateful components, are ES6 classes that extend from React.Component. They can hold and manage state and have access to the React lifecycle methods. Before hooks, class components were the only way to manage stateful logic in React applications. They provide a more structured approach to component creation and allow for more complex features, such as error boundaries and potentially better performance optimizations with shouldComponentUpdate.
In the current landscape of React development, the trend leans towards functional components for their conciseness and the power granted by hooks. However, class components are still a viable choice, particularly for those who are comfortable with object-oriented programming or are working on projects that were built before the advent of hooks.
Understanding when to use each type of component is key to writing effective React code. Functional components are generally used for presenting static information and managing UI state with hooks. In contrast, class components are often chosen for more complex state logic and lifecycle operations. However, with the continuous evolution of React and its capabilities, the lines between the two are increasingly blurring, offering developers flexibility and choice in their approach to building React applications.
5. Creating Your First React Component
Creating your first React component is a fundamental step in building React applications. Components are the building blocks of any React app, and understanding how to create them is essential.
To start, you’ll need to have a development environment set up with Node.js and npm (Node Package Manager). Once you have that in place, you can create a new React project by using the Create React App command-line tool. This tool sets up the necessary build configuration for you, so you can focus on writing React code right away.
With your React project initialized, you can start creating a new component. Components in React can be defined in two ways: as functional or class components. Functional components are simpler and are just JavaScript functions that return JSX, the syntax used for rendering UI elements in React. Class components, on the other hand, are ES6 classes that extend from React.Component and provide more features, such as local state and lifecycle methods.
For a functional component, the structure is straightforward. You simply create a function that returns JSX. Here’s a basic example:
function Welcome(props) { return <h1>Hello, {props.name}</h1>;}
This Welcome component takes in a name
property (prop) and renders an h1
element with a greeting. You can use this component in other parts of your app by importing it and including it in JSX like so: <Welcome name="Alice" />
.
If you need more complexity, such as handling state or lifecycle events, you might opt for a class component. This is how it looks:
class Welcome extends React.Component { render() { return <h1>Hello, {this.props.name}</h1>; }}
Like the functional component, this class component also returns an h1
element containing a greeting. However, it’s defined as an ES6 class that extends React.Component
.
Once you’ve defined your component, you can use it within other components or your application’s main file, typically App.js
. By importing the component and then using it as a custom HTML element, you can compose complex UIs from small, reusable pieces.
Remember that components should be named with PascalCase, meaning that each word in the name of the component should start with a capital letter. This convention helps to easily distinguish component names from HTML elements in your JSX.
React’s component-based architecture offers a modular approach to building user interfaces. By creating and combining components, you can efficiently develop and maintain complex applications. Start by crafting simple components, and as you become more comfortable with React’s concepts and syntax, you can explore more advanced features.
6. Component Properties (Props) Explained
Understanding component properties, commonly referred to as props, is essential when working with modern front-end frameworks such as React, Vue, or Angular. Props are how components communicate with each other, allowing for the passage of data from parent to child components. This data flow helps in building interactive and dynamic user interfaces.
Props are immutable within the child component, which means they should not be modified. Instead, they represent a ‘read-only’ contract between the parent and child. A well-designed component will use props to configure its behavior or to generate its rendered output. They are akin to function arguments, making components reusable and composable by providing different values for props in different instances.
When designing components, it’s crucial to define the types and expected structure of the props. This not only helps in preventing bugs but also improves the maintainability of the codebase by informing other developers of the intent and usage of the component.
To ensure that the component behaves as expected, default prop values can be specified. This avoids undefined prop errors and ensures that the component has all the necessary data to function properly.
Additionally, prop validation is a practice that enhances the reliability of the application. By specifying the required data type for each prop, you can catch type-related bugs during development, which can save time and reduce potential errors in production.
Remember, props are not just limited to simple data types like strings or numbers. They can also be functions, which is a common pattern for handling events and callbacks in a parent component. This allows child components to communicate back to the parent, ensuring that the data flow remains unidirectional and the architecture stays consistent.
By understanding and implementing props effectively, you can create highly modular and maintainable components that can be easily adapted and reused across different parts of your application. This leads to a more efficient development process and a more stable and scalable application architecture.
7. State Management in React Components
Understanding state management is crucial for developing interactive applications with React. State in React is an object that determines how that component behaves and renders. It can be as simple as a text string or as complex as an object containing a variety of data.
When dealing with state, the most basic approach within a React component is utilizing the useState
hook. This hook allows you to add React state to function components. By calling useState
, you create a single piece of state associated with that component. The state does not have to be an object — it can be a string, number, array, or any other type.
For class components, state management is handled through the this.state
property and the this.setState()
method. When state changes are enqueued, React updates the component, leading to a re-render to reflect those changes in the UI.
For more complex state management scenarios, especially when the state needs to be shared across multiple components, you might use React’s Context API or state management libraries such as Redux or MobX. The Context API allows you to share state across the entire app or parts of it without having to pass props down manually at every level.
Redux provides a centralized store for all your state and applies strict rules regarding how and when updates can happen, which can be beneficial for larger applications with more complex state changes. Redux’s principles of a single source of truth, state immutability, and predictable state transitions enforce a consistent approach to state management.
MobX offers a slightly different philosophy, using observables and actions to manage state. It allows for a more reactive style of coding, which can be less boilerplate-heavy than Redux, making it a good fit for those who prefer a more straightforward and less strict approach.
In summary, state management in React components involves understanding when to use local component state, leveraging React’s built-in hooks or lifecycle methods, and implementing more sophisticated tools like Context, Redux, or MobX for complex applications. Selecting the right approach to state management will depend on the specific needs of your application and your preferences in coding style.
8. Lifecycle Methods in Class Components
Lifecycle methods are crucial features of class components in React. They allow developers to tap into different phases of a component’s existence, from its creation to its destruction, and dictate how the component behaves during these phases.
Understanding lifecycle methods is essential for managing side effects, such as API calls, subscriptions, and manually manipulating the DOM. These methods provide hooks into specific points in a component’s lifespan: initialization, updating, and unmounting.
Initialization: This is the phase where the component is being set up and includes the constructor
method, where you can initialize state and bind event handlers. The componentDidMount
method is also part of this phase, which is a perfect spot for network requests and setting up any subscriptions.
Updating: Occurs when a component’s state or props change, prompting a re-render. Lifecycle methods involved in this phase include shouldComponentUpdate
, which determines if the component should re-render, componentDidUpdate
, which is called after the component updates, and can be used for operations like fetching new data based on the updated state.
Unmounting: The final phase of a component’s life is when it’s being removed from the DOM. Here, componentWillUnmount
is the key lifecycle method, providing an opportunity to clean up any subscriptions, timers, or pending network requests to prevent memory leaks.
By strategically leveraging these lifecycle methods, developers can ensure that the component behaves correctly throughout its life in the application, leading to more reliable and efficient UIs. It’s also worth noting that these methods are specific to class components, and React’s functional components use hooks like useEffect
to achieve similar outcomes.
Proper use of lifecycle methods can lead to significant performance optimizations and a smoother user experience, making them a fundamental aspect of React class component development.
9. Hooks: Modern State and Lifecycle Features in Functional Components
Hooks in React are powerful features that allow you to use state and other React features without writing a class. With hooks, you can encapsulate functionality in a more readable and maintainable way, which is especially beneficial for functional components that previously could only make use of state and lifecycle features by converting to a class component.
The useState hook is the first hook you’ll likely encounter and it’s what you’d use to add state to a functional component. By calling useState and passing the initial state, you receive an array with two elements: the current state value and a function that allows you to update it. This makes state management in functional components much simpler and cleaner.
For lifecycle capabilities, useEffect is the go-to hook. It tells React that your component needs to do something after render. useEffect can replicate the behavior of componentDidMount, componentDidUpdate, and componentWillUnmount lifecycle methods in class components. It takes two arguments: a function that runs after the DOM updates and a dependency array to control when the effect runs. By leveraging useEffect, you can perform side effects in your components, such as data fetching, subscriptions, or manually changing the DOM.
If you’re managing complex state logic, the useReducer hook can be a better choice. It’s similar to useState, but it accepts a reducer function and an initial state. This hook returns the current state and a dispatch function, allowing you to handle more intricate state-related logic.
To optimize performance and avoid unnecessary re-renders, you can use the useMemo and useCallback hooks. useMemo will memoize a computed value so that it doesn’t get recalculated on every render unless its dependencies have changed. useCallback, on the other hand, will return a memoized callback function, ensuring that functions passed down to child components don’t change unless necessary.
Another useful hook is useContext, which lets you tap into React’s Context API to share state across the component tree without having to pass props down manually. It’s especially useful for global state like themes, authentication status, or application-wide configurations.
Lastly, custom hooks are a powerful pattern that allows you to create reusable stateful logic. By extracting common behavior into a custom hook, you can share logic across multiple components and keep your codebase DRY (Don’t Repeat Yourself).
By utilizing these hooks, developers can write less boilerplate code, manage state and lifecycle events more intuitively, and ultimately create more maintainable and readable React applications. Leveraging these modern features in functional components leads to better scalability and easier refactoring, which aligns well with the principles of modern web development.
10. Handling Events in React Components
Handling events in React components is an essential aspect of creating interactive applications. React provides a synthetic event system that ensures consistency across different browsers. Events in React are named using camelCase, rather than lowercase, and with JSX you pass a function as the event handler, rather than a string.
To handle events in React, you typically create an event handler function within your component. This function is then passed to the element’s event property within your JSX template. For example, if you want to handle a click event on a button, you would create a handleClick
function and then pass it to the button’s onClick
property like so:
<button onClick={this.handleClick}>Click me</button>
It is important to note that in class components, event handlers need to be bound to the instance of the component to access this
. This binding can be done in the constructor or by using class field syntax to define your methods.
In functional components, which are often used with Hooks, you can define the event handler function within the body of the component. Since functional components don’t have an instance, you don’t need to worry about this
binding, which simplifies things considerably:
const MyComponent = () => { const handleClick = () => { // Event handling logic }; return <button onClick={handleClick}>Click me</button>;};
For events such as onChange
, onSubmit
, or onKeyPress
, you would follow a similar pattern, defining an event handler function that accepts an event parameter. This event parameter is a synthetic event that wraps the native browser event and has the same properties, such as event.target
that can be used to get the value of an input field, for example:
const handleChange = (event) => { console.log(event.target.value);};
It’s also possible to pass arguments to event handlers. This can be done by defining an arrow function that calls the event handler function with the arguments you want to pass:
<button onClick={(e) => this.handleClick(e, someArgument)}>Click me</button>
In this case, the arrow function creates a closure around e
and someArgument
, allowing you to pass them into handleClick
.
Remember to call preventDefault
on the event if you need to prevent the default behavior of the event in your handler function. This is particularly common in form submission handlers to prevent the form from being submitted and causing a page reload:
const handleSubmit = (event) => { event.preventDefault(); // Form submission logic};
By understanding and correctly implementing event handling in React, you can create rich, interactive user experiences. Keep in mind the importance of binding event handlers in class components, leveraging the simplicity of functional components, and using synthetic events to ensure cross-browser compatibility.
11. Adding Styles to React Components
When styling React components, you have several methods at your disposal, each with its own set of advantages. Understanding these methods allows you to effectively style your application in a way that enhances user experience and adheres to best practices.
Inline Styling is a technique where you apply styles directly to elements using the style
attribute. In React, inline styles are not specified as a string but as an object with camelCased properties. This method is useful for dynamic styles that depend on the component’s state or props, but it’s generally not recommended for most use cases because it can lead to code that’s hard to maintain and doesn’t separate concerns.
CSS Stylesheets are the traditional way to style web applications. You can create a .css
file and import it into your React component file. This approach is familiar to those with experience in web development, and it keeps your styles separate from your JavaScript code. However, styles defined in CSS are globally scoped by default, which can lead to conflicts if not managed carefully.
CSS Modules help solve the problem of global scope in traditional CSS. When you use a CSS module, class names and animation names are scoped locally by default. This means that you can use the same class name in different files without worrying about naming clashes. To use CSS Modules, you simply create a .module.css
file and import it into your React component using a specific syntax. This approach allows for modular and reusable styles.
Styled-components is a library for React and React Native that allows you to use component-level styles in your application. It utilizes tagged template literals to style your components. With styled-components, you can write actual CSS code to style the components without worrying about class name collisions. It also allows for the creation of dynamic styles that can be based on props, offering a powerful way to theme your components.
Emotion is another popular library similar to styled-components, but with a focus on performance and flexibility. Emotion provides two ways to style components: with styled components and with a CSS prop. Both methods allow for dynamic styling based on props and can be used to create highly performant, themable UIs.
Each method of adding styles to React components has its own use cases and benefits. Inline styles provide a quick way to apply dynamic changes, CSS stylesheets offer familiarity and global styling, CSS Modules introduce scoped styling, and styled-components and Emotion offer powerful and flexible ways to style components at the component level. When choosing a styling method, consider the scale of the project, performance requirements, and team preferences to select the approach that best fits your needs.
12. Component Composition and Reusability
Component composition and reusability are fundamental concepts in modern software development, enabling developers to create scalable and maintainable applications efficiently. These principles are particularly important in the realm of front-end development, where user interfaces are often built from modular, reusable components.
Firstly, component composition refers to the practice of building small, self-contained units of functionality that can be combined to create complex interfaces. This approach allows for a clear separation of concerns, making it easier to manage and update individual parts of the application without affecting others. Components can be nested within others, creating a hierarchy that reflects the visual and functional structure of the application.
Reusability, on the other hand, is the ability to use these components across different parts of an application or even across different projects. By designing components to be reusable, developers can save time and reduce the likelihood of bugs, as they can leverage well-tested units of code rather than repeating similar efforts multiple times.
To maximize reusability, components should be designed to be as generic as possible, which often means making them configurable with props or inputs that define their behavior and appearance. This way, a single component can serve multiple purposes, adaptable to various contexts and requirements. For example, a button component could be reused with different sizes, colors, and behaviors, depending on where it is used in the application.
It is also beneficial to follow a design system or component library that provides a consistent look and feel across the application. Such systems often come with a set of rules and best practices for component construction, ensuring that new components fit seamlessly with existing ones and adhere to the overall design language.
Effective component composition and reusability not only lead to a more efficient development process but also contribute to a more consistent user experience. By focusing on these principles, developers can build robust applications that are easier to test, maintain, and extend over time.
13. Managing Dependencies with PropTypes
Managing dependencies within a component-based architecture like React can be challenging, particularly when components grow in complexity. PropTypes is a library that assists in managing these dependencies by providing a way to explicitly list the props a component requires and the type of data each prop is expected to be.
Using PropTypes, developers can define a contract for their components. This contract specifies what properties the component expects and what type they should be. This is immensely useful for two reasons: first, it serves as a form of documentation for other developers who use the component, making it clear what props are needed and what their intended use is; second, it provides runtime type checking, which can catch bugs early by warning developers if a component is used incorrectly.
To use PropTypes, you need to first install the ‘prop-types’ package. Once installed, you can import it into your component file and define the propTypes object. Each key in this object corresponds to a prop your component receives, and the value is the expected data type, which can be one of several types such as PropTypes.string, PropTypes.number, PropTypes.bool, and so on.
PropTypes also allows for more complex structures, like object shapes, where you can specify the type of properties within an object prop, or arrayOf, for an array of certain types. Additionally, you can mark props as optional or required. If a required prop isn’t provided, PropTypes will log a warning to the console during development, which can be crucial for catching missing data before it leads to a runtime error.
By using PropTypes, you enhance the robustness of your code and the development experience. It’s a way to ensure that components are used as intended and that the data flowing through your application is valid. Although PropTypes does not provide type checking at compile-time like TypeScript, it is a lightweight solution that can still greatly improve the reliability and maintainability of your codebase.
14. Performance Optimization for React Components
Performance optimization in React is crucial for creating fast and responsive applications. One of the key aspects of optimizing React components involves minimizing unnecessary re-renders and computations, which can significantly improve the performance of your application.
To start, make use of React’s PureComponent
or React.memo
for class and functional components respectively. These tools perform a shallow comparison of props and state to determine if re-rendering is necessary. This means that if your component receives the same props and state, it won’t re-render, saving precious resources.
Another effective technique is to optimize your component’s state by breaking it down into the smallest possible pieces. This granular state management ensures that only the components relying on a particular piece of state re-render when that state changes, rather than the entire component tree.
State and props should also be managed carefully by keeping them as simple as possible. Avoid complex objects that can trigger deep comparisons and instead, opt for primitive types that are easier to compare and track for changes.
When dealing with lists or collections, the key property becomes particularly important. By providing a unique and stable key for each item, React can quickly identify individual elements, making the diffing process more efficient and preventing unnecessary re-renders.
Memoization is another technique that can be applied to prevent costly re-computations. By caching the results of functions and only recalculating when necessary, you can avoid redundant processing.
Lastly, consider the virtualization of long lists using packages like react-window
or react-virtualized
. These tools render only the items that are currently visible in the viewport, dramatically reducing the number of DOM nodes created and managed by React.
By following these strategies, you can enhance the performance of your React components, leading to a smoother user experience and a more efficient application. Keep in mind that optimization is a balance, and over-optimizing can lead to complexity and maintenance challenges. Therefore, always profile your application to identify bottlenecks before applying these techniques.
15. Testing React Components
Testing React components is a crucial step in ensuring the quality and functionality of your application. It helps detect bugs early, improves code quality, and ensures that components behave as expected after changes or updates. There are several testing methods and tools available to test React components effectively.
Unit Testing
Unit testing involves testing individual components in isolation from the rest of the application. This method is useful for verifying the functionality of components and ensuring that they produce the expected output for a given set of inputs. Tools like Jest, a delightful JavaScript Testing Framework with a focus on simplicity, and testing utilities like Enzyme or React Testing Library can be used for this purpose.
Integration Testing
Integration testing checks how multiple components work together. It’s a step up from unit testing and ensures that the combination of components interacts correctly. You might use the same tools as for unit testing but will focus on testing a group of components and their combined behavior.
Snapshot Testing
Snapshot testing is a way to ensure your UI does not change unexpectedly. By using Jest, you can take a ‘snapshot’ of a React tree with a particular state and then compare this snapshot to a reference image taken earlier to detect changes or regressions.
End-to-End Testing
End-to-end (E2E) testing involves testing the flow of an application from start to finish. It aims to replicate user behavior and ensures that the whole application functions correctly in a real-world scenario. Tools like Cypress or Selenium can be used to automate browser actions to perform these tests.
Performance Testing
Performance testing is essential to ensure that your React components render efficiently and do not cause any performance bottlenecks. Tools like Lighthouse and React Profiler can be used to analyze the performance of your components and find potential issues.
Accessibility Testing
Accessibility testing ensures that your application is usable by people with various disabilities. Tools like axe-core or pa11y can help automate the detection of accessibility issues in your components.
For all these methods, it’s important to have a good understanding of what you are testing and why. Writing good test cases and maintaining them as the application evolves is key to the effectiveness of your testing strategy.
Remember, the goal of testing React components is not only to find bugs but also to provide a safety net for future development, improve code quality, and ensure user satisfaction with your application. Regularly running these tests as part of your development process will lead to a robust and reliable codebase.
16. Advanced Patterns in React Components
Advanced patterns in React components enable developers to create more reusable, maintainable, and scalable applications. By understanding and applying these patterns, developers can enhance their components’ flexibility and simplify complex UI logic.
One of the advanced patterns is the Compound Components Pattern, which allows for a more declarative API when dealing with complex component structures. It involves creating components that work together by implicitly sharing state without requiring props drilling. This pattern is particularly useful in building UI kits or libraries.
Another important pattern is the Render Props Pattern. This pattern provides a way to share code between components using a prop whose value is a function. It’s a technique for sharing behavior across components and can be an alternative to using Higher-Order Components (HOCs).
Higher-Order Components are functions that take a component and return a new component. They are a form of component composition where the pattern is used to share common functionality between components without repeating code. HOCs can be used for a variety of tasks, such as handling state or modifying props before they are passed down to the wrapped component.
The Context API is another powerful pattern that facilitates the sharing of data without having to pass props down through every level of the component tree. With the Context API, developers can avoid props drilling and make data available to any component in the component hierarchy.
Hooks, introduced in React 16.8, offer a new approach to use state and other React features without writing a class. Custom Hooks are a pattern that allows developers to extract component logic into reusable functions. Hooks like useState
, useEffect
, and custom hooks enable developers to write more concise and modular code.
Lastly, the State Reducer Pattern gives more control over state updates in a component. It involves using a reducer function to manage state changes, which can be particularly valuable when dealing with complex state logic. This pattern allows for more predictable state transitions and can be combined with the use of the useReducer
hook for enhanced state management.
By mastering these advanced patterns, developers can build more robust and efficient React components. The key is to understand when and how to implement each pattern effectively to improve the architecture of React applications.
17. Common Pitfalls and Best Practices in Component Building
When building software components, it’s crucial to navigate common pitfalls and adhere to best practices to ensure scalability, maintainability, and performance. One common pitfall is over-engineering, which can lead to complex and rigid components. Instead, aim for simplicity in design, making components that do one thing well. This is often referred to as the Single Responsibility Principle.
Another pitfall is ignoring reusability. Components should be designed to be reused in different parts of the application or even across projects. This can be achieved by making them generic enough to handle various use cases, but not so generic that they become bloated and difficult to understand or use.
Avoid tightly coupling components to each other. Tightly coupled components can make the codebase fragile and difficult to refactor. Instead, use interfaces and dependency injection where possible to create a more modular and flexible architecture.
Neglecting accessibility is also a pitfall. Components should be built with accessibility in mind from the start, following guidelines such as the Web Content Accessibility Guidelines (WCAG). This ensures that your application is usable by as many people as possible, including those with disabilities.
Performance is another area where pitfalls can arise. Components that are not optimized can lead to slow and unresponsive applications. Make sure to consider performance implications such as rendering behavior and the use of computational resources. Techniques such as lazy loading, memoization, and avoiding unnecessary re-renders can greatly enhance component performance.
Testing is often overlooked but is a best practice that cannot be overstressed. Components should have unit tests to ensure they work as expected and integration tests to ensure they work within the larger application context. This not only helps catch bugs early but also provides documentation and makes future refactoring safer and easier.
Lastly, documentation should not be an afterthought. Well-documented components enable other developers in your team to understand and use them without having to dive into the implementation details. This includes not only comments in the code but also external documentation such as README files or documentation websites.
By avoiding these common pitfalls and incorporating these best practices into your component development process, you’ll create robust, efficient, and scalable software components that stand the test of time and changes in the development ecosystem.
18. Conclusion and Further Resources
For those who have followed along and absorbed the information presented, you now have a solid foundation to build upon your SEO knowledge and practices. To continue learning and stay abreast of the latest trends and algorithm updates, it is essential to regularly consult authoritative SEO resources.
Some valuable further resources include:
Google’s Webmaster Guidelines – These guidelines provide insights directly from the search engine giant, outlining best practices for creating a Google-friendly site.
Moz Blog – Moz offers a wealth of articles, whitepapers, and guides, as well as a thriving community for discussions on the latest in SEO.
Search Engine Land – For those seeking up-to-the-minute news on search engines and SEO, Search Engine Land is a must-follow.
SEMrush Blog – SEMrush offers not only a suite of SEO tools but also a blog filled with tutorials and case studies to help refine your SEO strategies.
Ahrefs Blog – Ahrefs provides deep dives into SEO topics, often accompanied by data and research to support their insights.
Yoast SEO Blog – Best known for their WordPress SEO plugin, Yoast’s blog is a great resource for understanding the technical aspects of SEO.
Backlinko – Brian Dean’s Backlinko is the place to go for actionable SEO techniques and in-depth strategies.
SEO by the Sea – Bill Slawski’s writings on search engine patents and theory provide a more academic perspective on SEO.
Online Courses and Webinars – Platforms like Udemy, Coursera, and LinkedIn Learning offer courses on SEO that range from beginner to advanced levels.
Industry Conferences – Attending SEO conferences such as SMX, BrightonSEO, or Pubcon can provide opportunities to hear from and network with industry experts.
Remember, the landscape of SEO is constantly changing. To maintain high rankings and keep your website visible to your target audience, it is crucial to stay informed and adapt your strategies accordingly. Always be testing and learning; SEO is an ongoing process rather than a one-time setup. Keep honing your skills, engage with the community, and leverage the wealth of knowledge available to ensure your online success.