Software Services
For Companies
For Developers
Portfolio
Build With Us
Get Senior Engineers Straight To Your Inbox

Every month we send out our top new engineers in our network who are looking for work, be the first to get informed when top engineers become available

At Slashdev, we connect top-tier software engineers with innovative companies. Our network includes the most talented developers worldwide, carefully vetted to ensure exceptional quality and reliability.
Build With Us
How to Build a Custom ERP System in Node.js/

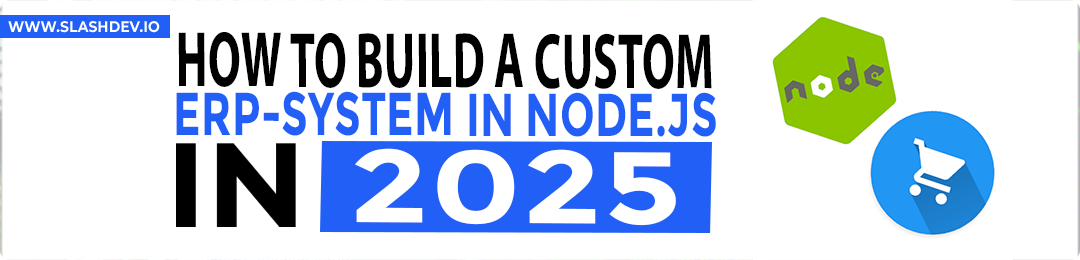
Building a custom ERP (Enterprise Resource Planning) system in Node.js can help streamline business operations by integrating different departments and managing a wide array of processes. A custom ERP system ensures that businesses can scale their operations, centralize their data, and improve productivity. In this article, we’ll walk through the process of building a custom ERP system using Node.js, from understanding its core features to implementing key modules.
1.Understanding the Core Features of an ERP System/
Before diving into the technical implementation, it’s important to identify the core features your ERP system should have. The specific features may vary based on your business requirements, but common modules include:
⦁ Inventory Management: Tracking stock levels, reordering products, and managing warehouses.
⦁ Order Management: Processing orders, tracking customer data, and handling shipping.
⦁ Customer Relationship Management (CRM): Managing client information, tracking interactions, and improving customer service.
⦁ Human Resources Management: Managing employee data, payroll, performance reviews, etc.
⦁ Accounting and Finance: Managing budgets, transactions, payroll, and invoices.
2.Choosing the Right Tools and Frameworks/
For building an ERP system, Node.js is an excellent choice. It’s fast, scalable, and has a vast ecosystem of libraries. Here’s an outline of some of the technologies you’ll likely use:
⦁ Node.js: A JavaScript runtime environment for building scalable applications.
⦁ Express.js: A minimal and flexible Node.js web application framework for building the backend.
⦁ MongoDB: A NoSQL database that pairs well with Node.js for handling large amounts of unstructured data.
⦁ Passport.js: An authentication middleware for Node.js to handle user login and sessions.
⦁ Socket.io: Real-time communication between the server and client, useful for order updates and notifications.
⦁ Step-by-Step Guide to Building Your ERP System/
Step 1: Set Up Your Development Environment
Start by setting up Node.js and your chosen database (such as MongoDB) on your system. Use npm (Node Package Manager) to install necessary dependencies such as Express.js, Mongoose (for MongoDB), Passport.js, and others.
Step 2: Define Your Database Schema
Your ERP system will need a database schema that can store data for each module. For instance, create schemas for inventory, orders, and employee details using Mongoose (if you’re using MongoDB).
Step 3: Building the Server
Use Express.js to create routes that handle the business logic. Start with basic routes such as creating a new order or fetching customer data.
Step 4: Implementing the User Interface
While the backend is critical, a good user interface (UI) is also essential for an ERP system. You can build the frontend using a framework like React.js or Angular.js to connect with your Node.js backend. The frontend will allow users to interact with the ERP system by adding or viewing data from different modules.
Step 5: Real-Time Communication
Incorporate real-time communication with Socket.io. This can be used for features like order status updates, instant notifications, or real-time collaboration between users.
Step 6: Authentication and Security
A secure ERP system is paramount, especially if sensitive data is being handled. Use Passport.js for authentication, ensuring users can securely log in to the system. Implement role-based access control to restrict access to different parts of the system based on user roles.
Step 7: Testing and Optimization
Once your ERP system is built, thorough testing is crucial. This includes functional testing to ensure that each module performs as expected and load testing to handle scalability. Optimize your Node.js application for performance, ensuring fast response times even when the system scales.
⦁ Additional Tips for Building a Custom ERP System/
⦁ Modular Design: Keep your ERP system modular. This way, you can add or remove features as the business grows or changes.
⦁ Scalability: Ensure your system is scalable from the start. Choose a cloud database (like MongoDB Atlas) and use cloud services for deployment (e.g., AWS or Heroku).
⦁ User Experience: Make the user interface as intuitive as possible, as ERP systems can be complex. Use UX best practices to design clean, navigable dashboards.
⦁ Backup and Disaster Recovery: Set up regular database backups and implement disaster recovery plans to protect against data loss.
⦁ Conclusion/
Building a custom ERP system in Node.js can significantly improve business processes by centralizing data, automating workflows, and providing real-time insights into company performance. By following the outlined steps, choosing the right frameworks and tools, and focusing on scalability, you can create a system tailored to your specific needs. Keep testing and optimizing your system for the best results, and you’ll have a powerful tool to manage your enterprise’s resources effectively.