Software Services
For Companies
For Developers
Portfolio
Build With Us
Table of Contents:
How to Build a Custom SaaS Application in Laravel/

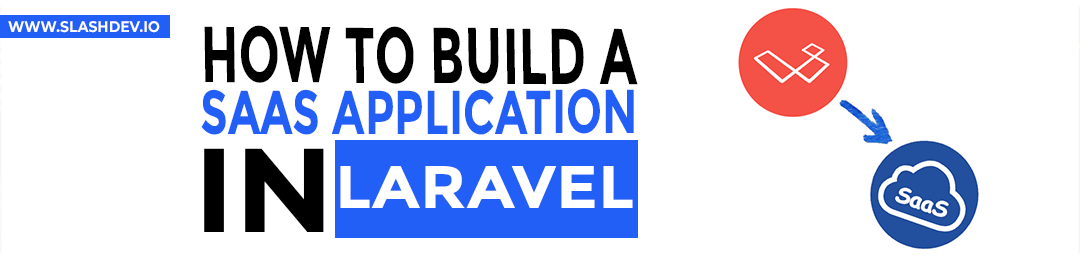
Building a custom Software as a Service (SaaS) application can be an exciting venture, and Laravel is one of the best frameworks to use for creating robust, scalable, and feature-rich applications. Laravel’s simplicity, elegant syntax, and developer-friendly tools make it a top choice for building web applications quickly and efficiently. In this guide, we’ll walk you through the essential steps for building a custom SaaS application using Laravel.
Step 1: Set Up Laravel
The first step to building a custom SaaS application is setting up a fresh Laravel project. If you haven’t already installed Laravel, start by setting up Composer (a PHP dependency manager) on your machine. Once Composer is installed, you can create a new Laravel project by running a simple command. This will generate a fresh Laravel project where you can begin building your application.
Step 2: Plan Your SaaS Application
Before diving into the code, it’s essential to plan your SaaS application. Determine the core features and the types of users that will use your app. For example:
⦁ User Registration and Authentication: Will you have different user roles? A multi-tenant system or just one account type?
⦁ Subscription Management: Will users pay for subscriptions? If so, how will you handle billing and payments?
⦁ Customization and Branding: Will users be able to customize their dashboards or interface?
Planning out these details in advance will help you determine the architecture and technology needed.
Step 3: Implement User Authentication
Laravel provides an excellent authentication system out of the box. For a SaaS application, you may need to customize authentication to support features such as multi-tenancy or role-based access control.
For basic user authentication, Laravel has a built-in system, but you might need to adapt it to fit your specific needs. For instance, if your app requires multi-tenancy (meaning each user has a different isolated environment), you can use packages that simplify this process. Multi-tenancy ensures that each user’s data and configurations are kept separate.
Step 4: Create Database Models and Migrations
Once your authentication system is in place, the next step is to build your database. Laravel’s Eloquent ORM makes this process straightforward. For a SaaS application, you’ll need to create models to represent different entities like User, Subscription, and Plan.
You can create a model and its corresponding database migration by running a simple command that generates both the model and the migration file. In the migration file, define the fields required for each model, such as name, email, password, and other business-specific data (e.g., subscription details). After defining the structure, run the migration to create the actual database tables.
Step 5: Integrate a Payment Gateway
For SaaS applications, managing subscriptions and payments is essential. Laravel has built-in integration with popular payment gateways like Stripe through the Cashier package.
Using Laravel Cashier, you can easily manage subscriptions, handle different payment plans, and integrate billing functionalities into your application. Stripe is one of the most commonly used payment providers for SaaS, but you can also explore other payment gateways based on your needs.
Once integrated, Cashier handles the subscription management, including payment processing, invoices, and subscription renewals, which are essential for any SaaS platform
Step 6: Build SaaS-Specific Features
At this stage, you can start building the features that make your application unique. For example:
⦁ Tenant Management: Implement multi-tenancy features if your SaaS app requires each client to have their own isolated space.
⦁ User Dashboards: Provide users with customizable dashboards where they can view and manage their data.
⦁ API Development: If your application will expose data or allow for integration with other services, building robust APIs using Laravel’s built-in tools is essential.
Consider building out features based on the needs of your users and your business model. Ensure that scalability is a priority, as SaaS applications often have high growth potential.
Step 7: Secure Your Application
Security is critical in any web application, but it’s especially important in SaaS applications where sensitive data (like payment information) is involved. Laravel offers excellent built-in security features, including protection against common vulnerabilities like CSRF (Cross-Site Request Forgery) and SQL injection.
Additionally, you should implement role-based access control (RBAC) to manage user permissions and restrict access to sensitive areas of the app based on user roles. This is especially important in SaaS platforms, where different types of users may have different levels of access.
Step 8: Test Your Application
Before launching your SaaS app, it’s crucial to test every aspect of your application thoroughly. Laravel’s built-in testing features allow you to write unit and feature tests to ensure that your app functions as expected. Testing will help you identify bugs and ensure that your users will have a smooth experience when using the app.
Use tools like PHPUnit and Laravel’s browser testing capabilities to simulate user interactions and validate the functionality of your application.
Step 9: Deploy and Scale
Once your application is ready, you can deploy it to a production server. Laravel is compatible with many hosting platforms, such as DigitalOcean, AWS, and Heroku. You should also consider implementing continuous deployment (CD) to streamline updates and improvements.
As your application grows, it’s essential to focus on scalability. Laravel’s built-in tools, like database migrations, queues, and caching, can help ensure that your application can handle increasing user loads and data volume.
Conclusion
Building a custom SaaS application in Laravel is an exciting journey that allows you to leverage one of the best PHP frameworks available today. By following these steps—setting up your Laravel environment, planning your features, implementing authentication and database models, integrating payments, and securing your app—you can create a powerful and scalable SaaS platform. With Laravel’s flexibility and developer-friendly tools, you’ll be able to build an application that can scale with your business and meet the needs of your customers.