Software Services
For Companies
For Developers
Portfolio
Build With Us
Get Senior Engineers Straight To Your Inbox

Every month we send out our top new engineers in our network who are looking for work, be the first to get informed when top engineers become available

At Slashdev, we connect top-tier software engineers with innovative companies. Our network includes the most talented developers worldwide, carefully vetted to ensure exceptional quality and reliability.
Build With Us
Concurrency in JavaScript: Techniques for Efficient Asynchronous Programming/

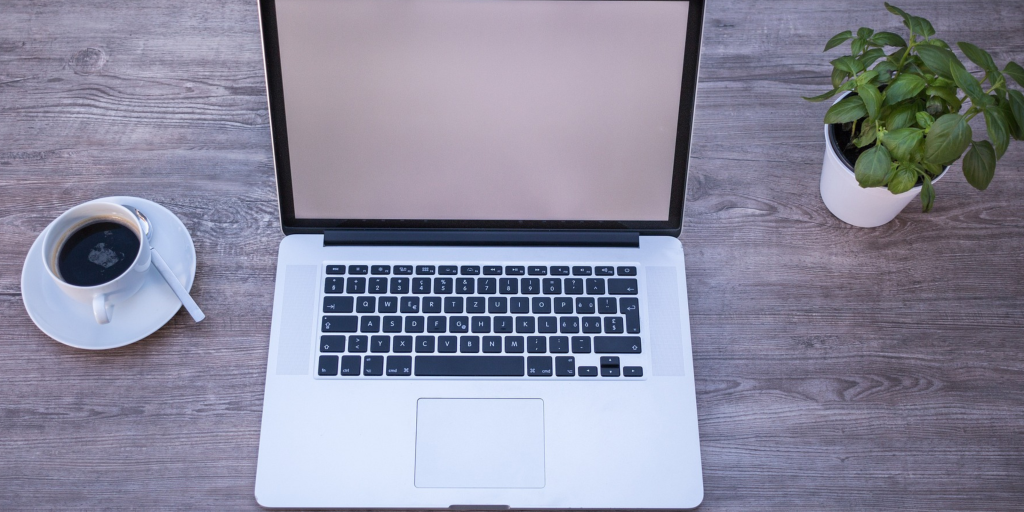
Introduction/
As web applications become more complex and user demands for seamless experiences grow, mastering concurrency and asynchronous programming in JavaScript has become crucial for developers. In this article, we will explore various techniques to achieve efficient concurrency, making your applications more responsive and scalable.
Understanding Concurrency in JavaScript/
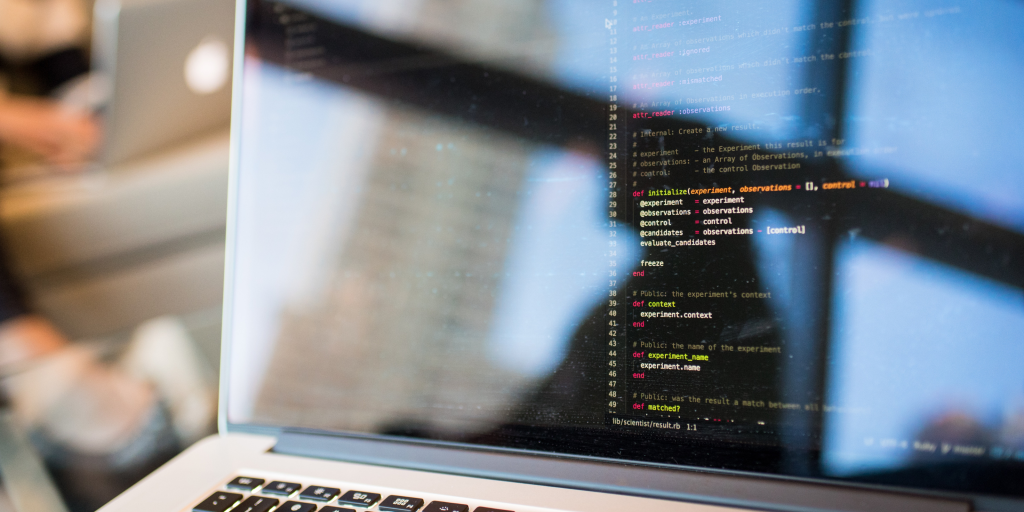
What is Concurrency?
We will start by defining concurrency in the context of JavaScript and how it differs from parallelism. Understanding the fundamental concepts of concurrency will set the foundation for exploring advanced techniques.
Event Loop
The event loop is the backbone of concurrency in JavaScript. We will dive deep into how the event loop manages the execution of asynchronous tasks and ensures non-blocking behavior.
Promises and Async/Await/
Promises
Promises have revolutionized asynchronous programming in JavaScript. We will explore how promises work, chaining multiple promises, and handling errors efficiently.
Async/Await
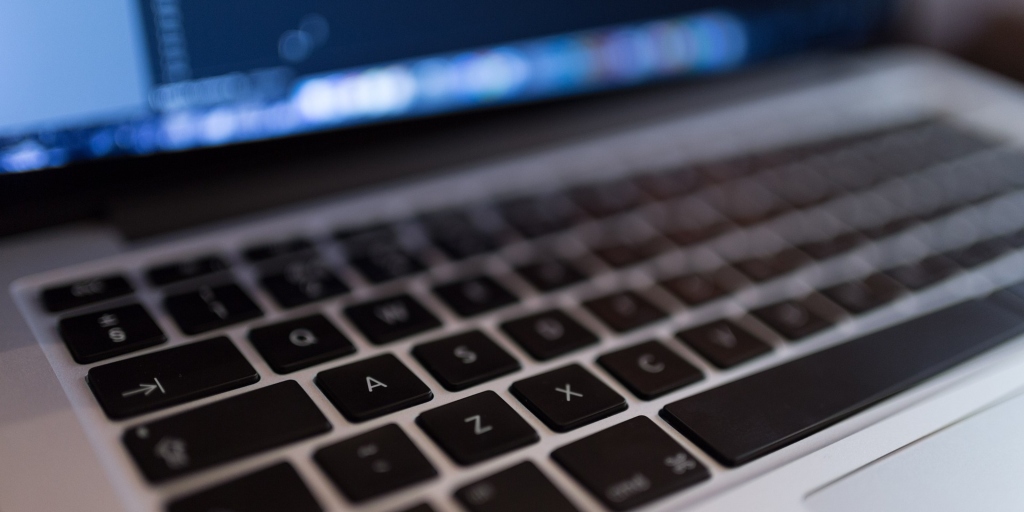
Async/Await is a powerful syntactical feature introduced in ECMAScript 2017. We will delve into how Async/Await simplifies asynchronous code and makes it look more synchronous.
Web Workers/
Introduction to Web Workers
Web Workers are a powerful mechanism for running scripts in the background, separate from the main UI thread. We will discuss how Web Workers can be used to achieve true parallelism and improve application performance.
Shared Workers
Shared Workers allow communication between multiple instances of a web application. We will explore how Shared Workers facilitate intercommunication and data sharing between tabs or windows of the same application.
Service Workers/
Leveraging Service Workers
Service Workers enable powerful features like offline support and push notifications. We will explore how Service Workers work, and how they can enhance the overall user experience by caching resources and providing real-time updates.
Background Sync
Background Sync is a feature offered by Service Workers that allows data synchronization even when the user is offline. We will discuss how Background Sync can be implemented to ensure data integrity.
Parallel Array Processing/
Parallelism with Web Workers
Web Workers can be used for parallel array processing to optimize tasks that involve heavy computation. We will demonstrate how to leverage Web Workers to process arrays in parallel, reducing overall execution time.
Concurrency Patterns and Best Practices/
Throttling and Debouncing
Throttling and debouncing are essential techniques for controlling the frequency of function execution. We will explore how to implement these patterns to optimize performance and prevent excessive function calls.
Concurrent Data Fetching
Managing multiple data fetch requests can be challenging. We will discuss strategies to handle concurrent data fetching, avoiding over-fetching and under-fetching data.
Real-time Data with WebSocket/
Introduction to WebSockets
WebSockets offer bidirectional communication between the client and the server, facilitating real-time data updates. We will explore how WebSockets can be used to create real-time applications.
Implementing a Real-time Chat Application
As a practical example, we will build a real-time chat application using WebSockets, demonstrating the power of real-time data transmission.
Conclusion/
Mastering concurrency and asynchronous programming in JavaScript is crucial for creating efficient and responsive web applications. By understanding the event loop, utilizing promises, and leveraging advanced features like Web Workers and Service Workers, developers can optimize application performance and deliver exceptional user experiences. For more valuable insights and resources on JavaScript development, visit slashdev.io, a platform dedicated to empowering developers with the latest tools and knowledge.